PHP: Switch Statement
PHP Switch Statement Tutorial. Learn how to work with Switch statements in PHP, and about using Switch vs If Statements.
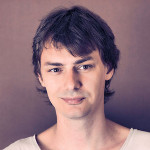
By. Jacob
Edited: 2019-10-07 14:40
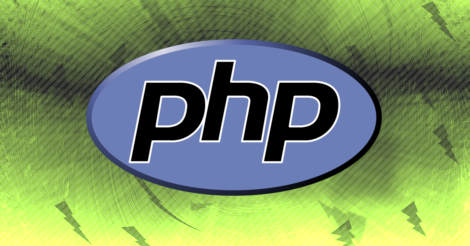
The Switch is similar to using PHP IF statements, but works a bit differently. The expression is only evaluated once, while in an if statement, it will be evaluated again and again. Hence why a switch may be faster when used inside loops.
While a switch statement in theory should be faster than an if statement, this is not necessarily the case, depending on circumstances. Under certain circumstances, such as when using a switch inside a loop, you might notice some difference in speed.
To create a switch, simply do like in the below example:
switch ($page) {
case "frontpage":
// Do stuff here
break;
case "blog":
// Do other stuff here
break;
}
PHP Switch Example
In he below example, we will "switch" to the matching code block depending on the value of the $i variable.
switch ($i) {
case 0:
echo "i equals 0";
break;
case 1:
echo "i equals 1";
break;
case 2:
echo "i equals 2";
break;
}
It is also possible to use a switch with string values, this may be accomplished like demonstrated in the below example:
switch ($input) {
case "Beamtic":
echo "Beamtic Is cool!";
break;
case "Rocking":
echo "Beamtic Rocks!";
break;
case "Beaf":
echo "Beamtic is totally beaf!";
break;
}
How a switch works
The switch is read one line at a time, and stops executing when a case matches the input, at which point the matching code block will be executed. In this example, the case must match the contents of the $input variable.
Its important that you do not forget to include a break;, otherwise PHP will also execute the code inside the following case "code block". You may however intentionally chose to leave it out in some circumstances, and that is totally fine – as long as you know what you are doing.
switch ($input) {
case "Frontpage":
include 'Frontpage.php';
break;
case "Rocking":
echo "Beamtic Rocks!"; // Will not get executed if Beaf
case "Beaf":
echo "And Beamtic is totally beaf!"; // Gets executed if Rocking
break;
}
PHP Switch vs If statements
It is pretty much down to personal preference, and while there might be a slight performance difference, its not of any significance compared to other points of optimization.
Some sources state that the switch statement is faster, while others find if statement to be the fastest. I have yet to find a good explanation on when to use a switch rather than if's.
In reality, the reason why there may be differences in results, might be due to different PHP versions. Another reason could be the way the switch is used in people's code, because, as mentioned, it should be faster in theory.
For most developers it is more a question about personal preference. So you are generally free to choose whatever coding style you want. Points of optimizations are probably best looked for elsewhere, such as with your database queries and file system access.
Tell us what you think: