Setting Request Headers With AutoIt
How to set the request headers when performing HTTP requests.
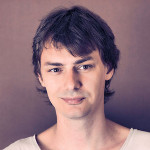
By. Jacob
Edited: 2021-04-12 08:56
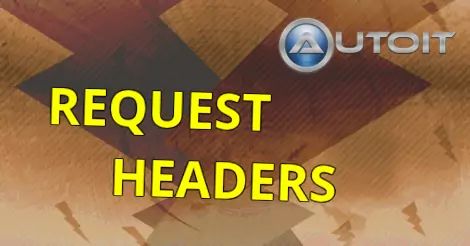
In this tutorial you will learn how to set Request Headers and handle cookies using the Winhttprequest.5.1 object with AutoIt; this is useful for purposes such as changing the user-agent and accept-encoding request headers.
The following example shows how to use the SetRequestHeader method of the Winhttprequest.5.1 object to configure request headers of a HTTP request:
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("GET", "https://beamtic.com/api/user-agent", False)
$oHTTP.SetRequestHeader("User-Agent", "User-Agent=Mozilla/5.0 (Windows NT 6.1; WOW64; rv:12.0) Gecko/20100101 Firefox/12.0")
$oHTTP.SetRequestHeader("Referer", "http://beamtic.com/")
$oHTTP.Send()
You may want to mimic the user-agent of a popular browser as this may help you to avoid getting blocked by some sites.
User agent lists:
Handling Cookies with AutoIt
Cookies are also delivered with the SetRequestHeader Method, this is useful to know if you are making a program that should be able to login on a website.
But before you can deliver cookies, you will need to have some cookies to be delivered. When making HTTP Requests, any cookies sent by the server, will be available trough the GetAllResponseHeaders method, after the request has been sent.
$oHTTP.Send()
$HeaderResponses = $oHTTP.GetAllResponseHeaders()
The response headers contains more than just the Cookies, so you will need to figure out a way to pick just the cookies. One way to do that is demonstrated below:
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("GET", "https://beamtic.com/api/request-headers", False)
$oHTTP.SetRequestHeader("User-Agent", "User-Agent=Mozilla/5.0 (Windows NT 6.1; WOW64; rv:12.0) Gecko/20100101 Firefox/12.0")
$oHTTP.SetRequestHeader("Referer", "http://beamtic.com/")
$oHTTP.Send()
$HeaderResponses = $oHTTP.GetAllResponseHeaders()
; Handle Cookies
$array = StringRegExp($HeaderResponses, 'Set-Cookie: (.+)\r\n', 3)
$cookies = ''
for $i = 0 to UBound($array) - 1
; Add all cookies to a single string, and then clean it up.
$cookies = $array[$i] & ';'
; Getting the name of the Current Cookie
$cookname = StringRegExpReplace($cookies, "([^=]+)=.+", "$1")
; Removing parts we do not use..
$cookies = StringRegExpReplace($cookies, "( path| domain| expires)=[^;]+", "")
$cookies = StringRegExpReplace($cookies, " HttpOnly", "")
$cookies = StringRegExpReplace($cookies, "[;]{2,}", ";")
; Save the cookies to .txt files
; Delete the file if it already exists
FileDelete ("Cookies\" & $cookname & ".txt")
$file = FileOpen("Cookies\" & $cookname & ".txt", 1)
If $file = -1 Then
MsgBox(0, "Error", "Unable to open file: " & $cookname)
Exit
EndIf
FileWrite($file, $cookies)
FileClose($file)
Next
You should now be able to deliver the cookies back to the server when performing subsequent requests. To deliver the cookies, you can create a function to check the directory to see if there is any cookies to be delivered:
Func DELIVER_COOKIES()
; Search for files in the cookie directory and add them to the cookies variable, seperated by ",,,"
$search = FileFindFirstFile("Cookies\" & "*.*")
If $search = -1 Then
return 'none'
EndIf
$cookies = ''
While 1
$file = FileFindNextFile($search)
If @error Then ExitLoop
$result = StringInStr($file)
If $result > 1 Then
$cookies = $cookies & ',,,' & $file
EndIf
WEnd
FileClose($search)
$cookies = StringTrimLeft($cookies, 3) ; Fix the string
; Split the cookies into an array
; then read the files one by one, and add their contents to the cookie variable
$array = StringSplit($cookies, ',,,', 1)
$counter = 1
$cookie = ''
While $counter <= $array[0]
;MsgBox(0, "File: ", $array[$counter])
$file = FileOpen("Cookies\" & $array[$counter], 0)
If $file = -1 Then
Exit
EndIf
$cookie = $cookie & FileRead("Cookies\" & $array[$counter], -1)
FileClose($file)
$counter = $counter + 1
WEnd
Return $cookie
EndFunc
The above function returns the contents of the cookies as a string, which can be included trough the SetRequestHeader method. When sending the cookies back to the server, simply do like this:
$Cookie = DELIVER_COOKIES()
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("GET", "https://beamtic.com/api/user-agent", False)
; Deliver the cookies returned by DELIVER_COOKIES()
$oHTTP.SetRequestHeader("Cookie", $Cookie)
$oHTTP.Send()
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think: