Check if string contains a substring with PHP
Check if a string contains a substring using strpos and regular expressions.
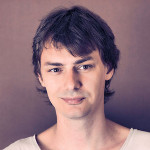
By. Jacob
Edited: 2020-07-27 17:51
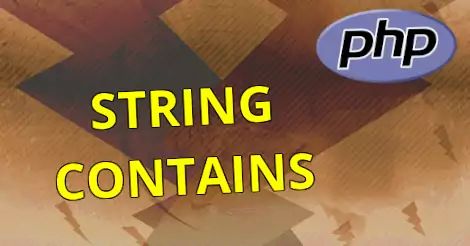
Performing a if string contains check in PHP can be done in several ways, one of the best ways is by using strpos(), but it is also possible to check for more complex patterns with regular expressions.
In programming, this problem is usually described as the needle in haystack problem. You may know this figure of speech from everyday life. The source string is the haystack, and the substring is the needle we are searching for.
A common situation where you need to find a string in another string, is when validating e-mail. Either you can use strpos to check for the existence of a "@" character, or you can create a regular expression to perform a more complex validation. PHP has a build-in way for e-mail validation, so creating your own is likely a bad idea.
The below is a simple check using strpos:
if (false !== strpos('string contains PHP', 'PHP')) {
echo 'PHP was found in string';
} else {
echo "Not found!";
}
From PHP 8 onwards, we can use the str_contains function:
if (str_contains('string contains PHP', 'PHP')) {
echo 'PHP was found in string';
}
Strpos
The strpos function will either return false if substring is not found, or a numeric value representing it's position if it is found. This is why we use the "!==" operator, which loosely means "not equal to". A value of "0" would also be understood as false, so doing a "!=" comparison will not work. The "!==" operator will however, as it makes PHP do an an exact comparison.
$source_str = 'beamer@beamtic.com';
$substring = '@';
if (false !== strpos($source_str, $substring)) {
echo 'Found it';
} else {
echo "Not found!";
}
Note. Again. If $substring is found in $source_str, and it is located in the beginning (the 0'th position), it will return this position. Hence we use "!==" or "<>" as the comparison operator:
$source_str = 'beamer@beamtic.com';
$substring = '@';
if (false !== strpos($source_str, $substring)) {
echo 'Found it';
} else {
echo "Not found!";
}
Note. Use stripos for a case-insensitive version of strpos.
Strstr
While Strstr can be used for the same purpose, it is discouraged by the PHP manual, stating that strpos is both faster and uses less memory:
If you only want to determine if a particular needle occurs within haystack, use the faster and less memory intensive function strpos() instead.
Using strstr is similar to using strpos:
if (false !== strstr($source_str, $substring)) {
echo 'Found!';
} else {
echo 'Not found!';
}
Note. strchr is an alias for strstr
Regular expressions
We can use a Regular expression (regex or regexp) when we need to match multiple parts in a string. One place where they are useful, is when we want to validate user input from submit forms.
To use a regex we can use the preg_match function.
Below is a very simple match, which can also be performed using stripos:
if (preg_match("/neeDle/i", "There's a small needle in a haystack")) {
echo "Found!";
} else {
echo "Not found!";
}
The i at the end of the pattern /neeDle/i makes the regex perform a case-insensitive match, so in the above case "needle", with small letters, will be match.
There is a more advanced function, preg_match_all, which allow us to work with all the matches in a string. For example, one place were is useful, is when you work with HTML documents, and you wish to find all tags of a certain type, lets say headings (h1-h6).
You could use this to create a "table of contents" list for an article, using the heading titles as anchor text on your links. In the following example, I will show how this is done.
$table_of_contents = '';
$source_string = '<body> code here....
<h1 id="section1">Some HTML fragment</h1>
<p>Example paragraph</p>
<h2 id="section2">Subsection to h1</h2>
<p>Second example paragraph</p>
<h2 id="section3">Subsection to h2</h2>
<p>Third example paragraph</p>';
preg_match_all("|<h[^>]+>(.*)</h[1-6]+>|i",
$source_string,
$headings, PREG_SET_ORDER);
$match_count = count($headings);
for ($i = 0; $i < $match_count; $i++) {
$table_of_contents .= "\n ". '<li><a href="#section'.$i.'">' . $headings["$i"][1] . '</a></li>';
}
$table_of_contents = '<ol>' . $table_of_contents . "\n". '</ol>';
echo $table_of_contents;
The "\n" creates a linebreak. I only included it to beautify the code a bit.
Depending on your own HTML coding style, you may need to modify the above to make it work.
Tell us what you think: