Splitting strings into arrays
How to break down strings into smaller parts using PHP.

Edited: 2020-01-10 02:49
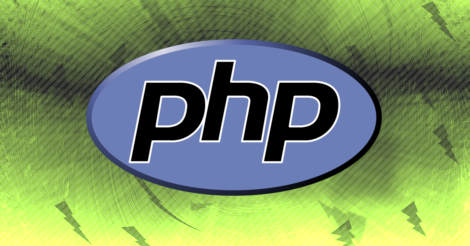
The preg_split and explode functions are used to take a string apart, break it into smaller pieces, and put it into an array. The preg_split function allows the use of complicated string manipulation with regular expressions to divide a string into smaller chunks, while the explode function only uses a single delimiter. Which one to use depends on the circumstances, but explode will most likely be the easiest to use.
See also: Arrays in PHP
Using explode
The explode function works by breaking down a string into smaller strings, and placing them in an array for easy access. The string to be broken down is often just a comma separated list, but you do not have to use a comma, a longer delimiter will work as well.
$full_string = "part1 part2 part3 part4 part5 part6";
$parts_array = explode(' ', $full_string);
echo $parts_array[0]; // Part1
echo $parts_array[1]; // Part2
In the above example a single blank space character was used as the delimiter.
When the string is broken down into smaller parts, each part will be available in the $parts_array array, this can then be worked with using a foreach type of loop construct:
$full_string = "part1 part2 part3 part4 part5 part6";
$parts_array = explode(' ', $full_string);
foreach ($parts_array as &$part) {
echo $part . "\n"; // Outputs all the parts one by one followed by a linebreak
}
Using preg_split
The preg_split function can be used to perform splitting operations that are more complicated, and allow us to split by a regex pattern rather than a string delimiter.
When splitting a list of user entered keywords, you will often have to account for the possibility that the user misses some commas, and mixes in spaces. This cab be done with the following code:
$keywords_array = preg_split("/[\s,]+/", "Movies, music books");
print_r($keywords_array);
The reguler expression we used in this example will look for one or more whitespace characters and commas (\s,), meaning that the string will be broken on either comma or whitespace characters, as well as when there are multiple of them.
Tell us what you think: