Escaping Backslash in PHP
Backslash needs to be tediously excaped in PHP, both when uccuring inside your PHP script, and when used with preg_replace.
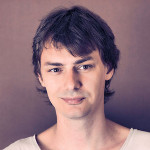
By. Jacob
Edited: 2020-12-26 18:03
I recently had a very strange problem where a literal backslash would not work within PHP strings under some circumstances, even when using single quotes. This is because backslashes have special meaning when used in the code of PHP scripts.
If a backslash is located at the end of a single quoted string, the backslash needs to be escaped. For this reason, it is probably best to always escape your backslashes, since it will avoid confusion in the code.
For example, I created a regular expression to replace both forward slash and backslash from file paths, including duplicate slashes like "//", however, I kept getting errors because the backslash was not properly escaped.
With preg_replace, backslash needs to be double escaped:
$str = 'c:\\\\this\\\\test\\\\';
echo preg_replace('#[/\\\\]+#', '/', $str);
Apparently you need to escape the backslash twice within a regular expression, hence the double escape "\\\\".
The output is:
c:/this/test/
Not escaping a backslash can result in an error like:
PHP Parse error: syntax error, unexpected end of file in backslash.php on line 5
Regular expressions and backslash
The problem with backslash appears to have two levels to it. First, we must escape backslashes that are declared inside the code of our PHP scripts. Secondly, we will also need to escape literal backslashes used in regular expressions.
The problem with regular expressions exists in two places — both in the regular expression pattern, and in the replacement string!
This is because backslashes have special meaning in both places.
When performing a replacement operation, we can use preg_quote for the pattern, but this can not be used for the replacement string; instead we need to escape each literal backslash with another backslash.
$replacement_id = 'REPLACEMENT_ID';
// Escape backslashes in PHP script (First level)
$unescaped_string = 'c:\\test\\testing\\some-file.txt';
// Escape backslashes in the replacement string (Second level)
$escaped_string = str_replace('\\', '\\\\', $unescaped_string);
$new_html = preg_replace('|{$replacement_id}|', $escaped_string, $html);
Note that this would prepare the the replacement string to use with preg_replace — but in most cases you can probably just use str_replace or substr_replace instead.
Replacing a string within some HTML
Let us say you want to replace a unique string within some HTML code, and you just want to do a "drop-in" replacement, without modifying the replacement string; as it turns out, this sort of thing is easier to do with str_replace, since it avoids having to escape backslashes at the second level; you do however still need to escape literal backslashes that you declare as strings within your PHP script:
$html = '<div>REPLACEMENT_ID</div>';
$replacement_id = 'REPLACEMENT_ID';
$replacement = '<pre>c:\\test\\some-file.txt</pre>';
$html = str_replace($replacement_id, $replacement, $html);
echo $html;
Output:
<div><pre>c:\test\some-file.txt</pre></div>
Why use replacement ids?
Sometimes you just need to do drop-in replacements, but I also realize most of you probably do use back-references with preg_replace; if you understand the problem, applying the fix to your own code should not be very hard..
In this tutorial I am focusing the problem caused by having backslashes in your pattern or subject strings, and not how to write the patterns themselves. The drop-in replacement was a simple way to demonstrate this problem.
To learn how to write basic patterns for replacing strings, see this tutorial: How to replace parts of a string with str_replace and preg_replace
Links
- Strings - php.net
Tell us what you think: