Arrays in PHP, syntax, iteration, and search of arrays
This tutorial shows how to make- and work with different types of arrays in PHP using different syntaxes.

Edited: 2021-03-07 10:03
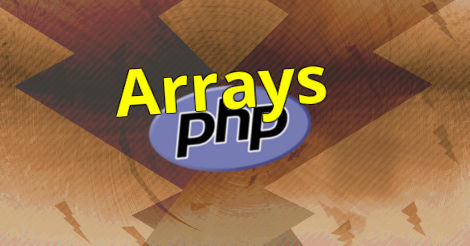
Arrays makes it easy to associate elements (values) with keys in a type of "list". The individual elements can then be used later in a script.
Arrays are useful in many situations, but a common use is when you need to work dynamically on a set of elements in a list. They are also easier to pass on to functions than variables are, since you can supply the elements in any order you like, while parameters needs to be supplied in a fixed order.
There is three different ways to write an array, the two ways is by using array() and [] syntax, which are functionally identical.
The short-hand syntax works with square brackets []:
$myArray = [
'full_name' => 'Jacob',
'email' => '[email protected]'
];
The same can be written using array() syntax:
$myArray = array(
'full_name' => 'Jacob',
'email' => '[email protected]'
);
The third way to code an array is also the most readable; it works by first declaring the array, and then assigning values by key reference:
// Declare the variable as an array
$my_array = [];
// Assign some elements to the array
$my_array['name'] = 'Hansen';
$my_array['birth_year'] = '1975';
Creating arrays
An easy way to create PHP arrays for beginners that is easy to remember, is by defining one element at a time:
$MyArray = array();
$MyArray[] = 'Peter';
$MyArray[] = 'John';
$MyArray[] = 'Matthew';
The above code would automatically assign a key to each value we place in the array, and it is easy to add more elements as needed. We may also assign a key manually. An array key can either be an integer or a string, in the above example a integer value is assigned for each element, beginning with "0".
Tip. If a string key is very long, it may sometimes help to use a hash of the string instead. Though, there is no limit on allowed characters.
Assigning a key manually is easy, simply do like this:
$MyArray = array();
$MyArray['name'] = 'Peter';
$MyArray['phone'] = '20170315';
$MyArray['email'] = '[email protected]';
Another common way to create an array, which may be somewhat harder to remember:
$MyArray = array(
"name" => "Peter",
"phone" => "20170315",
);
Determining the length of an array
Counting elements in an array can be done using the PHP count function:
$aCoolStuff['php'] = "PHP is Cool";
$aCoolStuff['css'] = "CSS is Cool";
$aCoolStuff['html'] = "HTML is Cool";
echo count($aCoolStuff); // 3
If the second parameter, mode, of the count function is used, the function can be made to work recursively, allowing us to count elements in multidimensional arrays:
$aMyPHPArray[0] = "Key1 content";
$aMyPHPArray[1] = array(
'SubKey1' => 'SubKey1 content',
'SubKey2' => 'SubKey2 content');
$aMyPHPArray[2] = "Key2 content";
$n = count($aMyPHPArray, 1); // Number of elements in array
echo $n; // returns 5
Iterate over arrays
Looping through arrays can be done using a while loop, after first determining the length of the array. Or, if you do not know the length of the array, by using a foreach loop.
A foreach loop is likely the simplest way to iterate over arrays in PHP, and it can also be used on objects.
$MyArray = array();
$MyArray[0] = 'One';
$MyArray[1] = 'Two';
$MyArray[2] = 'Three';
foreach ($MyArray as &$value) {
echo $value . '<br>';
}
To get both the key and the value:
foreach ($array as $key=>$val) {
echo $key .'->'.$val.'<br>';
}
A while loop may be used when the length of the array is known, and a $counter variable is used to manually keep track of the position in the array.
$MyArray[0] = 'One';
$MyArray[1] = 'Two';
$MyArray[2] = 'Three';
$n = count($aMyArray); // Count the number of Elements in array
$counter = 0; // Initialize the counter variable
while ($counter < $n) {
echo $aWords["$counter"] . '<br>';
++$counter; // Increment the counter by 1
}
Searching for keys in arrays
To check for the existence of certain array keys, we may use the isset, and array_key_exists functions.
A simple way to check if a given key exists, is to use PHP's isset function. I.e.:
if (isset($MyArray["2"])) {
echo 'Found';
} else {
echo 'Not Found';
}
Note. isset will not work with certain key names. NULL and 0 will return false, even when they do exist.
Another way to check for keys, which avoids the problem with isset, is with the array_key_exists function:
if (array_key_exists(3, $MyArray)) {
echo 'Found';
} else {
echo 'Not Found';
}
Search array for values and return keys
You can search an array for a value using PHP's in_array, while array_search can be used to return the key name of an element. To check for the existence of a string or number, simply do like this:
$aMyTest = array("MyTest1", "MyTest2", "MyTest3", "MyTest4");
if (in_array("MyTest2", $aMyTest)) {
echo "Found MyTest2" . "<br>";
}
if (in_array("MyTest5", $aMyTest)) {
echo "Found MyTest5";
} else {
echo "MyTest5 not found in array!";
}
You may obtain the key name itself using array_search:
$aMyTest = array();
$aMyTest[0] = 'red';
$aMyTest[1] = 'green';
$aMyTest[2] = 'blue';
$key = array_search('green', $aMyTest); // Outputs: 1
echo $key;
Sneaky use of Arrays
As you may know, using isset() is generally the fastest way to check if a variable exists. We can use this knowledge to our advantage when designing our array structure.
The most obvious place we can use this is when checking for the existence of array keys. But, if we instead use the elements as keys, we can also use this in certain places to drastically speed up our application.
For example, you should generally avoid searching for elements in an array because it can be very slow on big arrays. Instead, you should try to design your arrays so that you can use isset() or $some_array["$key"] to find what you are looking for. An easy way to do this is like so:
$to_check_for = 'One';
$some_array = array();
$some_array['One'] = true;
$some_array['Two'] = true;
$some_array['Three'] = true;
if (isset($some_array["$to_check_for"])) {
echo 'Found!';
exit();
}
In PHP, arrays are very flexible when it comes to allowing special characters to be used as keys, so you should not worry that including a space or line break will break your application.
Doing it this way can be many times faster than looping through an array to search for a value, and it is also extremely useful when filtering duplicates from an existing array. For example:
$some_array = array();
$some_array[] = 'One';
$some_array[] = 'One';
$some_array[] = 'One';
$some_array[] = 'Two';
$unique_array = array();
// Easily remove duplicates
foreach ($some_array as &$value) {
$unique_array["$value"] = true;
}
// Output the content of the unique array
foreach ($unique_array as $key => $value) {
echo $key . "\n";
}
Tell us what you think: