Centering Elements in CSS, Including Links and Buttons
There are several different techniques that can be used to center an element in its container, both vertically and horizontally; this tutorial will take you through some of the easiest techniques to use.
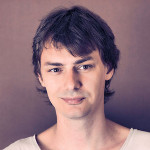
By. Jacob
Edited: 2021-02-13 15:15
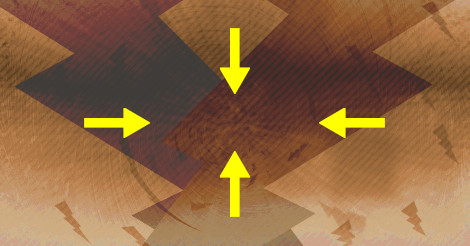
If you want to center a button in a div, it is actually not much different than if you wanted to center any other HTML element. The technique is almost the same, regardless of the element that you want to center. The only gotcha you need to be aware of, is that elements need to have a set width that is less than the containing element — but this makes sense, since block-elements will automatically take up the full width of the container — so how do we best decide a width that fits around the content?
We can do this in two ways. We can either experiment with a relative unit like em or rem, trying to determine a fitting value, or we can use fit-content as the width-value:
div {margin:0 auto;width:fit-content;}
A value fit-content is somewhat well supported; annoyingly, it does not appear to work well in Firefox, so what can be done instead? The easiest solution would be to use the vendor specific rules, if they are available. fit-content is currently experimental in FireFox, but seems to work fine with its vendor-specific equlivant, -moz-fit-content, so we can simply declare multiple width properties:
div {
margin:0 auto;
width:fit-content;
width:-moz-fit-content;
}
Vendor-specific values are ignored by other browsers.
Beware that in order to center an inline element such as span, a, input, or button you also need to define it as display: block; before the element complies with your decree:
button {display:block;margin:0 auto;width:8rem;}
Result:
There are also other techniques that are better supported by browsers; these will be covered in the following sections.
Using display:flex; to center elements
An alternative to the margin-based technique would be to define the containing element as a flexbox, and then throw in justify-content:center;. Example below:
.container {
display:flex;
justify-content: center;
}
And the HTML:
<div class="container">
<button>click me</button>
</div>
Using display:inline-block; and text-align
If an inline element is defined as an inline-block, then it will behave more as a block element, which allows you to set a width and a height on the element. In addition, it will also be affected by text-align, which makes it easy to center the element in its container — the only disadvantage is that you sometimes need to reset the text-alignment of the child-elements. Example below:
.container {
text-align: center;
}
.container button {
display: inline-block;
text-align:left;
}
Vertically centering an element
In the previous sections you saw how it was possible to center an element horizontally, but what if you want it to be vertically centered? You probably rarely have a need for this, nevertheless, it is sometimes a desirable design goal.
Since the early days of CSS, we have never had a decent way to center an element in its container vertically. With flexbox, this has become much easier. We can now do like this:
.container {
display:flex;
align-items: center;
justify-content: center;
height: 15rem;
}
.insider {
height: 5rem;
}
align-items: center; is the code-piece that causes the vertical alignment.
And the HTML:
<div class="container">
<div class="insider"><p>Hallo World</p></div>
</div>
Result:
Hallo World
Tell us what you think: