Combining JavaScript Files With Babel
How to combine multiple JavaScript files into a single file using Babel.
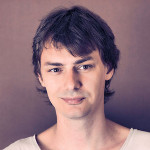
By. Jacob
Edited: 2019-08-25 12:31
I was working on optimizing my JavaScript resources on Beamtic, and in this connection I decided to use Babel to both minify and combine my JavaScript files. I could easily have made my own PHP script to combine the files, but since I also needed minification, I decided I might as well learn to use Babel, and even benefit from their many plugins, in case I needed them.
The thing is, once your JavaScript reach a certain size, you will likely start to organize it better. In my case, I am getting started with OOP in JavaScript, and I plan to make many classes (one class per file typically) that I can load as needed. It is simply not practical to load these individually via <script> elements, since this would slow down a site considerable.
Babel not only allows us to combine (Aka. concatenate) our JavaScript files into a single file, which was the requirement in my project, it also allows us to minify and convert our code so it will work on older browsers.
I am not usually a fan of supporting older browsers, perhaps because I am traumatised by IE6 and my whole process of learning how to code, many years back. But, ensuring browser support manually can be very time consuming. With JavaScript, I just want the ability to use latest features, and Babel makes this easy without me having to worry about browser support.
On your local development machine, after installing npm and babel locally, and also after setting up the project directory via npm init, all I had to do was to run this command from a terminal:
npx babel src --out-file script-compiled.js
It takes all the files in the src directory and combines (concatenates) them into a single file. I can then link this file via <script> in the HTML as I normally would.
I can easily configure things so I can work on a local development machine using the plain JS files, this way I do not have to worry about "compiling" my JavaScript for the live site until I am ready to do so.
Minification with Babel's minify plugin
If you want to use the minification plugin, it needs to be installed with:
npm install babel-preset-minify --save-dev
After this, you can either use the the plugin by including it as a preset via the command line, or you can create a configuration file that automatically loads the preset when you run babel in your project. I have so far tried to do both.
To use the preset directly from a terminal, you can do like this:
npx babel src --presets minify --out-file script-compiled.js
Writing the preset with the command is a bit annoying, so instead you might want to create a configuration file for your project.
To use a configuration file, create a file named .babelrc in the root folder of your project, then paste the following into the file and save it:
{ "presets": [ "minify" ] }
You can then compile your scripts by writing something like:
npx babel src --out-file script-compiled.js
Tell us what you think: