Creating New DOM Elements in JavaScript
In this Tutorial I am going to show how to create new elements in JavaScript, and how to add various types of content.
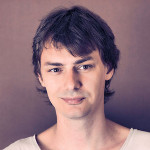
By. Jacob
Edited: 2020-09-26 11:57
To create an HTML element in plain JavaScript, we can either use the DOM createElement() method, or we can choose to simply insert the HTML directly.
There is no one way which is considered "the best", and which one to use depends mostly on personal preference, coding style, and project requirements.
On one hand, it can be argued that DOM methods are safer to use in the long run, since the element will be closed automatically, and adding attributes is also less prone to typos and other errors that tend to sneak in over time. On the other hand, it can also be argued that using innerHTML requires less code, and is therefor faster and cleaner to work with.
To create a new element using DOM methods, and fill it with some content, we may use the following:
let newElement = document.createElement('div');
newElement.innerText = 'Hallo World';
document.body.appendChild(newElement);
The above code can be further minimized to:
let newElement = document.createElement('div').innerText = 'Hallo World';
document.body.appendChild(newElement);
If we use innerHTML instead, we can do things like making text bold or italic using b and i in the content:
let newElement = document.createElement('div');
newElement.innerHTML = '<b>Hallo World</b>';
document.body.appendChild(newElement);
Adding child elements to a new element using DOM
When we use HTML DOM methods, we can create multiple new elements using the createElement() method, and then "append" the elements to other "abstract" elements using the appendChild() method.
Note. In this case, "abstract" simply means that we have not yet added the element to the HTML DOM.
In the following example code, to keep things simple, I will just create two abstract elements and append one to the other, then finally add the elements to the actual HTML DOM:
// Create the HTML Elements
let newDiv = document.createElement('div'); // The parent element
let newP = document.createElement('p'); // The child element
// Include some text in the paragraph element
newP.innerText = 'This is the paragraph';
// Append the child paragraph to the parent div
newDiv.appendChild(newP)
document.body.appendChild(newDiv);
Understandably, some web developers find it easier to just use innerHTML.
innerHTML vs innerText
The difference between innerHTML and innerText is that innerHTML will allow you to work with the raw HTML content of a node, while innerText only allows you to set and retrieve the plain text content of a node.
When using innerText to retrieve content, HTML elements will automatically be removed, while innerHTML will preserve them.
When using innerText you do not have to handle special characters, since they will be understood as literals by the browser. However, when using innerHTML, you will need to replace literals with their HTML entities. I.e. "<" and ">".
Which one to use depends mostly on project requirements and personal preference.
Tell us what you think: