Responsive HTML Video With CSS
Learn how to make responsive HTML videos the right way.
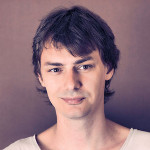
By. Jacob
Edited: 2019-10-13 06:02
Making a video responsive with CSS is actually very simple. In practice, it is no different than making an image responsive, and you should rarely think of it any differently – there is no need to make things more complicated.
Thanks to HTML5 (or the Living Standard), it is now easy to embed videos in the HTML, and stream the videos directly from a web page, without having to use third party players. In the past, you would typically rely on Flash Players to stream video, but this is no longer necessary!
For the sake of simplicity, I will focus on Chrome and Firefox in this tutorial. Both browsers support the .mp4 format, which is good for streaming.
The HTML should look like this:
<video>
<source src="your_video.mp4">
Your browser does not support the video tag.
</video>
While the CSS to make the video responsive should look like this:
video {
width: 100%;
min-width: 300px;
}
If you are skilled with coding already, then the above will be all you need, otherwise you may want to read on about the more complex issues with responsive images and video.
Making videos responsive
Normally you will just need to set width:100%;, and leave the height at auto (default) in responsive designs, but if you also want to handle content jumping problems, then you also need to define the height of the media files you are including.
The easiest solution may be to simply define the original width and height of the media inline, via the width and height HTML properties, while taking care of the rest in your CSS or JavaScript. If this is not possible, you should probably change to another CMS, or improve your code so it automatically fetches the width/height from the files before including them in the HTML.
Make video fill the screen
This is not always possible, since a video typically needs to maintain its aspect ratio, which is the relationship between the videos width and height. If you just stretch the video to fill the screen both horizontally and vertically, the video could become unwatchable, and this is also generally considered a bad practice among professional designers.
What can you do instead then? You can choose to "zoom" the video inside a container element with overflow:hidden;. But, this is extremely bad, since it would cut away parts of the picture in order to make it fill the screen vertically. Zooming might also makes the video extra "grainy" depending on resolution.
If the video is designed carefully, some cutting and zooming might be tolerable. But, a better solution would be to make videos for the different aspect ratios you target, and then load the one that best fits the given screen via CSS Media Queries
Hiding an element with display:none; also means that the browser will not load the content of the element until you decide to unhide it via a media query.
The absolutely best solution, in many situations, is not to do anything. Simply accept the fact that your video will not be a perfect fit for all devices and screen sizes.
If the aspect ratio of the video matches the screen, then you may be able to make the video take up all available space. Remember to also remove margin and padding, this is typically done via a global reset like this:
* {
margin:0;
padding:0;
}
All you really need is to give the video a width of 100%. The default value of height is auto, meaning that the height will be calculated automatically.
video {
width: 100%;
min-width: 300px;
}
The width and height attributes
It has long been recommended to always include a width and height attribute for images and videos, and this might be why many tutorials include the width and height attributes in code examples. But, it is not a requirement, and if you understand the problem it is trying to solve, then go ahead and use CSS if you wish.
The reason for including them is mainly that having a width and height in pixels, either in your critical CSS or via HTML attributes, will prevent a page from "jumping" as it loads the content.
However, as I mentioned, you can also use CSS for this purpose. The problem with using CSS, loaded from a "critical" StyleSheet, is that it scales poorly with lots of images of different dimensions. You would have to update the StyleSheet every time you add a new image!
Also, if a StyleSheet is not loaded before the content starts loading, then the styles might not be applied on time, and we will still have the jumping problem as the page DOM continues to load.
Note. Using render-blocking CSS, the above scenario is unlikely afaik.
There is also this idea that images and video is "content", and therefor dimensions should be declared inline instead of via style elements (be it external or embedded). I do not agree with this logic – but the problem is that browsers can not know the dimensions of the image before loading it, which makes width/height attributes the best solution.
It also does not solve the problem for responsive images and videos, as they will be using a relative length unit, which still causes jumping, even when the attributes are used!
The jumping problem
It gets more complicated when dealing with responsive images and videos, because you can not predict the height of the content easily, resulting in some jumping as they load. In many cases, this is tolerable, and not that big a deal. However, an easy fix would still be nice.
One solution is to use the aspect-ratio property in combination with width/height attributes:
img, video {
aspect-ratio: attr(width) / attr(height);
width: 100%;
}
There is even talk of implementing this in browsers. This way an image or video will automatically have its dimensions calculated based on the width and height attributes, if they are used, without developers having to do anything in their own CSS.
This method is very simple to implement, and gracefully degrades on older browsers.
Tell us what you think: