TypeError: Cannot read properties of undefined (reading _wrapper)
This problem can happen when you use an event handler in Twig, but forget to add- or place your function in the wrong location in your index.js.
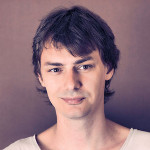
By. Jacob
Edited: 2023-12-31 05:59
I am currently working on a plugin for Shopware's administration back-end, and when I wanted to add a few onLoad things to the root element, I figured I would just try and add them to a function in the computed section of my index.js – this caused the following error:
TypeError: Cannot read properties of undefined (reading '_wrapper') at ar (vue.esm.js:7630:13) at ht (vue.esm.js:2238:7) at Array.sr (vue.esm.js:7643:3) at A (vue.esm.js:6369:60) at vue.esm.js:6248:9 at A (vue.esm.js:6374:29) at vue.esm.js:6248:9 at A (vue.esm.js:6374:29) at vue.esm.js:6248:9 at A (vue.esm.js:6374:29)
Apparently this can happen when you use an @event in twig, and do not add a function to the methods section of your index.js. I now have the following, which solved the problem (note the methods section):
const { Component } = Shopware;
const { Criteria } = Shopware.Data;
import template from './my-custom-page.html.twig';
import './my-custom-page.scss';
Component.register('my-custom-page', {
inject: ['repositoryFactory'],
template,
metaInfo() {
return {
// ...
};
},
data() {
return {
// ...
}
},
computed: {
// ...
},
methods: {
mainStart() {
setTimeout(() => {
console.log('do stuff here');
}, 1000);
return true;
},
}
})
Interestingly enough, the code in the function was working in spite of being erroneously placed in the computed part rather than adding it in methods. It was something else and entirely unrelated that was breaking because of my error, and the obscure error message was the only thing indicating something was wrong. Good luck finding it I guess?
In order to have the function called on the onLoad event, I would simply use @onload on my wrapper element inside my twig file. E.g:
<div class="fe-newsletter-product-selector" @onload="mainStart">
{# A bunch of more stuff here... #}
</div>
Tip. Functions placed in the methods: { ... } sections will be callable in your twig file with {{ myFunctionName() }}, and similarly so for variables placed in the data() { ... } section. E.g. {{ myVariableName }}
Note. This error is not to be confused with this other common Shopware "error": Cannot read properties of undefined (reading 'data')
Final notes
Of course, a better way of having something happen on the initial page load in vue.js is to rely on the its inherent reactiveness. I did not know that when I first wrote this. To be clear. You probably want to create a "watcher" to watch a property for changes – a watcher can execute a piece of code whenever a property is updated.
Another nice feature of vue is v-model on input elements for example. You can use v-model to directly bind a data () { ... } property to an input element – whatever the user enters in the input will be automatically stored in the data property, and vise-versa. This will also work with certain other "user-input" elements.
Using v-model with Computed properties is also an option.
Finally, if you have some data that you need loading in your .twig, and you are not sure whether it is available yet, you could add a v-if="element.config.myDataProperty" in your twig element, that way the element will only be rendered when the myDataProperty becomes available.
Tell us what you think: