How to Create Loops in AutoIt
How to use while, for, and do until loops, and how to loop through arrays and object in AutoIt.
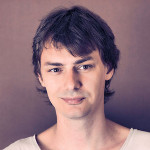
By. Jacob
Edited: 2021-02-14 02:40
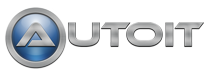
In different languages, Loops are often very alike. Usually they will need a counter, and a way to increment the counter on each loop completion.
In AutoIt we have the while and for loops, which you may know from other languages. Still, the syntax might be a little different than what you are used to. In addition, we also got the Do Until.
Here is how to create a while loop:
$counter = 0
While $counter <= 5
MsgBox(0, "Value of $counter is:", $counter)
$counter = $counter + 1
WEnd
Do Until Loops
To create a Do Until loop, we will first delacre the count variable, this will be incremented on each loop execution.
Local $i = 0
Next we'll create the actual Loop, the until statement says that the loop should continue until the $counter equals 10, which will never happen as it is currently. I.e.
Do
Until $counter = 10
For the counter to actually count, we will need to increment it, which can be done with the following piece of code.
$counter = $counter + 1
Full script:
Local $counter = 0
Do
MsgBox(0, "Value of $i is:", $i)
$counter = $counter + 1
Until $counter = 10
While Loops
The while loop begins with the while keyword, followed by a statement – while the statement is false, the loop will continue running.
$counter = 0
While $counter <= 5
MsgBox(0, "Value of $counter is:", $counter)
$counter = $counter + 1
WEnd
As you can see, the syntax reminds a lot of that in the Do Until example.
For Loops
The For loop may feel a bit different, or harder to use for some – but as a rule of thumb, you should just remember that the statement is written immediately after the For keyword. Also, the counter is automatically incremented after each iteration, which avoids having to do it inside the loop block itself, and that somewhat simplifies the code.
For $counter = 1 to 10
MsgBox(0, "Counter: ", $counter)
Next
Iterating Over Arrays and Objects
You can also use the For to loop through arrays and objects, this can be done with the In keyword.
Local $aArray[4]
$aArray[0] = "Hallo"
$aArray[1] = 0
$aArray[2] = 1.9233
$aArray[3] = "Another String"
Local $string = ""
For $element In $aArray
$string = $string & $element & @CRLF
Next
MsgBox(0, "For..IN Arraytest", "Result is: " & @CRLF & $string)
You Iterate an object almost the exact same way as you would be handling an array. The following example will output your currently open windows in a message box.
Local $oShell = ObjCreate("shell.application")
Local $oShellWindows = $oShell.windows
If IsObj($oShellWindows) Then
$string = ""
For $Window In $oShellWindows
$string = $string & $Window.LocationName & @CRLF
Next
MsgBox(0, "", "Your Current Open Shell Windows:" & @CRLF & $string)
Else
MsgBox(0, "", "You have no open Shell Windows.")
EndIf
Tell us what you think: