Sending HTTP Requests with AutoIt
This Tutorial will focus on post requests in AutoIt, using the Winhttprequest.5.1 object.
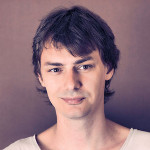
By. Jacob
Edited: 2021-04-12 08:53
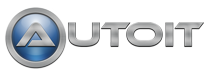
This AutoIt tutorial covers the topic of HTTP requests. While there are other ways to perform the requests, this tutorial focuses on the Winhttprequest.5.1 COM object. The Winhttprequest.5.1 COM object is also available in other programming languages, reading the tutorials and references for those can also help you understand how it works for AutoIt.
Whenever you use a web browser to visit a website, a HTTP request is performed to fetch the web page. It is possible to perform such HTTP requests from within AutoIt, and in many other scripting and programming languages.
The advantage of sending HTTP requests rather than automating a browser, is that you do not have to overcome challanges figuring out ways to interact with the browser in different screen resolutions. It also allows the script to run silently in the background, without disturbing the user.
There are two parts of a HTTP Request, the head, and the body. The head contains all the headers and the response code, while the body contains the content of the resource (I.e.: text/html, Images, and so on).
GET Requests
To make the Request, we first need to create a new instance of the Winhttprequest.5.1 object – if you are new to objects, try to think of it as a real object that you can turn, flip, and work with in your hands – in a much similar way, you will be able to do the same with an object in a programming language.
The object is really just a variable that you would name as any other variable.
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
The next thing to do, is to give the object the URL that you want to fetch, this can be done with the Open method.
$oHTTP.Open("GET", "http://beamtic.com/", False)
Finally we can send the Request, this is being done with the Send method.
$oHTTP.Send()
This would give us the following AutoIt Script.
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("GET", "http://beamtic.com/", False)
$oHTTP.Send()
The full script should look similar to the below.
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("GET", "http://beamtic.com/", False)
$oHTTP.Send()
$oReceived = $oHTTP.ResponseText
$oStatusCode = $oHTTP.Status
If $oStatusCode == 200 then
$file = FileOpen("Received.html", 2) ; The value of 2 overwrites the file if it already exists
FileWrite($file, $oReceived)
FileClose($file)
EndIf
POST Requests
When sending a HTTP POST request with AutoIt, all you have to do is to use the POST value in the Open method – the open method is also briefly mentioned in the last tutorial.
$oHTTP.Open("POST", "http://beamtic.com/Examples/http-post.php", False)
After doing this, you will also need to provide the data to be posted – this data will consist of the fields in the form, as well as their values – the data is stored as a string inside a variable, which is then passed on to the send method.
$sPD = 'name=Jacob&bench=150'
$oHTTP.Send($sPD)
Each form field is separated by an ampersand (&) in the $sPD variable – knowing this, adding additional fields won't be much trouble. Two fields are added in this example, a field called name and one called bench, these fields will be picked up by the script which handles the form submissions.
When you submit a form from your browser, the Content-Type field will be filled automatically if its missing – you need to fill it out manually when using AutoIt. The default value for forms is application/x-www-form-urlencoded. You can use the SetRequestHeader method to set the Content-Type field.
$oHTTP.SetRequestHeader("Content-Type", "application/x-www-form-urlencoded")
beamtic has a php form script at http://beamtic.com/Examples/http-post.php – this should make it easier to learn about http requests. You can try to create a request without the bench field, and see what happens.
The below script will perform a HTTP post request for the URL: http://beamtic.com/Examples/http-post.php – it will catch the response code, and save the body response to a html file for examination.
; The data to be sent
$sPD = 'name=Jacob&bench=150'
; Creating the object
$oHTTP = ObjCreate("winhttp.winhttprequest.5.1")
$oHTTP.Open("POST", "http://beamtic.com/Examples/http-post.php", False)
$oHTTP.SetRequestHeader("Content-Type", "application/x-www-form-urlencoded")
; Performing the Request
$oHTTP.Send($sPD)
; Download the body response if any, and get the server status response code.
$oReceived = $oHTTP.ResponseText
$oStatusCode = $oHTTP.Status
If $oStatusCode <> 200 then
MsgBox(4096, "Response code", $oStatusCode)
EndIf
; Saves the body response regardless of the Response code
$file = FileOpen("Received.html", 2) ; The value of 2 overwrites the file if it already exists
FileWrite($file, $oReceived)
FileClose($file)
Getting the Response Code
We can use the response code from the server to make decisions in our script via simple If statements. The status code can be retrieved using the Status property.
$oStatusCode = $UrlCheck.Status
The above saves the status code into the $oStatusCode variable. The response code is just a number, such as 404, or 200 which tells if the resource was found on the server.
$oStatusCode = $oHTTP.Status
If $oStatusCode == 200 then
; Do some stuff here
EndIf
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think:
Very easy to use and very well explained.
The only challenge is the code breaks at: $oHTTP.Send($sPD) if the server goes down and I have no clue how to handle it. Can you help or guide on what to do in such scenario?
Thanks.