If, Else, Else If in JavaScript
Learn how to use if statements in JavaScript to perform conditional execution of code.
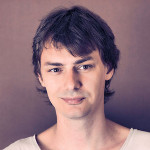
By. Jacob
Edited: 2021-02-05 23:59
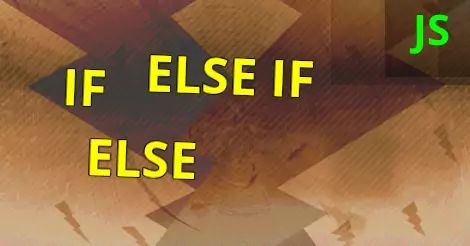
In JavaScript, it is possible to use if statements to perform conditional execution of code. This means that we can examine the state or condition of "something", typically a variable, and then decide what needs to be done based on the contents or "state" of the variable.
To give you a specific example, we could have a HTML input field where the user enters their name, and then use JavaScript to check if the field matches a specific name when the submit button is pushed.
A simple comparison can be done using comparison operators such as equal to == and not equal to !==. We can use these special operators in our if statements by using the syntax in the following examples.
A simple example of how to compare two variables:
if (inputName == knownName) {
console.log('The name matched');
}
Syntax
Compare a variable with a string:
if (inputName == 'Jacob') {
console.log('The name matched');
}
Compare a variable with a number (Integer):
if (inputInt == 88) {
console.log('The number matched');
}
In addition, we may also use greater than (>) and less than (<) to compare the size of two numbers:
if (inputInt < 33) {
console.log('The number was less than 33');
}
Adding an Else If statement:
if (inputInt == 88) {
console.log('The number matched: 88');
} else if (inputInt == 77) {
console.log('The number matched: 77');
}
We can as many else if's as we want.
Finally, if none of the statements matched, we can also ad an else block at the end:
if (inputInt == 88) {
console.log('The number matched: 88');
} else if (inputInt == 77) {
console.log('The number matched: 77');
} else {
console.log('No match was found');
}
Comparing user input from HTML forms
One place where we often need to compare values, is when accepting input from a user.
In order to use if statements to check input by users, we could use a HTML form, since a form has better accessibility than div elements. Do not worry, it is possible to style a form with CSS so it looks exactly as you want it!
Remember to also use for attributes on your labels to maximize the accessibility of your form. A for attribute on a label should correspond with an id on a input element.
A working example is included below:
The JavaScript code for this example:
let exampleForm = document.querySelector('#dk_exampleForm');
exampleForm.addEventListener('submit', event => {
event.preventDefault();
let inputName = document.querySelector('#dk_exampleFormInput').value;
let knownName = 'Jacob';
if (inputName == knownName) {
document.querySelector('#dk_exampleFormResult').innerHTML = "The name matched";
} else {
document.querySelector('#dk_exampleFormResult').innerHTML = "Name not found!";
}
});
Note. While it is possible and common to use a div as a button, it is hard to make it accessible, so it is not recommended; button is usually a better choice.
We can use preventDefault to stop the form from being submitted the normal way, and then we can instead handle it from JavaScript. In some cases it may be a good idea to still handle the form on the server-side when someone has JavaScript disabled in their browser. If you also handle the form on your server, then it should not be much extra trouble to make it work for users with JavaScript disabled.
The HTML for the form used in the above example, without all the CSS styling:
<form id="dk_exampleForm" method="post">
<label for="dk_exampleFormInput">Type your name:</label>
<input type="text" id="dk_exampleFormInput" name="user_name" value="" style="padding:0.2rem;">
<input type="submit" value="submit" id="dk_exampleFormButton" style="margin:1rem;padding:0.5rem;">
<p><b>Result:</b></p>
<div id="dk_exampleFormResult" style="margin:1rem;padding:0.2rem;"> </div>
</form>
Tell us what you think: