querySelector vs getElementById, which is best and why
Should you use querySelector or getElementById?; querySelector is more flexible, and able to perform more complex selections.
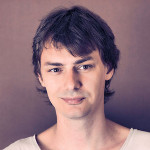
By. Jacob
Edited: 2021-03-07 10:58
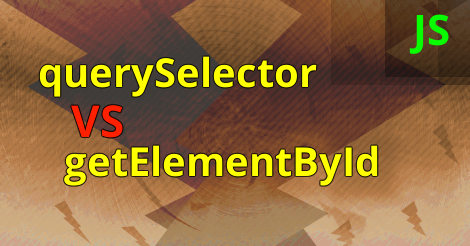
You will often need to perform more complex selections on your HTML, and that is where querySelector can be more useful; using it consistently can also make your code easier to read for other coders.
Both these methods can be used to select elements based on a unique ID or class, and are able to perform millions of selections per second; Keep in mind that performance may depend largely on the system and Browser of your users. On my old i3 laptop, I was able to do just about 800.000 selections per second in Google Chrome 88, and querySelector was only 6% slower — that is really fast!
Which one to use when selecting by id mostly comes down to personal preference. Of course, the query* functions are newer, and able to perform more complex operations using CSS selectors.
The thing with getElementById is that it only allows to select an element by its id. The querySelector method can be used in other situations as well, such as when selecting by element name, nesting, or class name.
In other words, the main benefit of using querySelector or querySelectorAll is that we can select elements using CSS selectors, which gives us a uniform way of dealing with element selection, and that makes it a preferred way of selecting elements to many developers — myself included!
Selecting an element by id is done simply by using the hashtag (#) followed by the element name, similar to how you would do it in CSS:
let element = document.querySelector("#unique_id");
You can also select elements that belong to a certain class, but you may consider using querySelectorAll instead, since this method allows you to select multiple elements.
When selecting classes, remember the dot (.) in front of the class name:
let element = document.querySelector(".element_class");
getElementById vs querySelector
querySelector is a more powerful tool when selecting elements since it allows for more complex selections; it could easily end up becoming the standard way of performing selections on elements, since it is now supported by all major browsers.
Read: can I use: querySelector and querySelectorAll? - caniuse.com
For consistency, it is probably best to choose one and stick with it throughout your scripts. It just looks less messy. Of course, if you are comfortable using the older methods, there is probably no reason to stop — it is just a different tool that works slightly differently.
Consider the fact that younger developers might work on the code, and they might get unnecessarily confused if they encounter inconsistencies such as multiple ways of doing the same things. This require extra mental energy on processing the code, and not only due to confusion but also due to people getting annoyed over how "messy" the code is.
Note. A simple search and replace is often enough to ensure consistency in your code.
The querySelector method is supported by all major browsers while getElementById can be used if support for really old browsers is needed. If you use a tool like Babel to support older browsers, then it may be irrelevant, since the newer functions can be converted to backwards compatible code when you compile your script.
How to use querySelectorAll
When selecting multiple elements with querySelectorAll, you will usually loop over the elements using a forEach loop.
It is also possible to use other types of loops, but for the sake of simplicity let us stick with the forEach in this tutorial.
let all_images = document.querySelectorAll(".article_image");
all_images.forEach(image => {
console.log('image: ', image);
});
Note we used console.log() above; This will log a message to your browser-console. You can view this message by opening developer tools in your browser (ctrl + shift + i in Chrome).
Alternatively, you can also use alert().
Finally, keep in mind you can also preview the result of querySelectorAll() using console.table():
let all_images = document.querySelectorAll(".article_image");
console.table(all_images);
getElementsByClassName and querySelectorAll
There is a major difference between how querySelectorAll and getElementsByClassName works – the former being the more powerful one.
Keep in mind, querySelectorAll is supported by all modern browsers where getElementsByClassName is better suited for when you want to support older browsers.
var elements = document.getElementsByClassName("myClassName");
Selecting elements by class name will create an object of the selected elements, you can loop through this object to handle the selection:
elements.forEach(element => {
console.log('element: ', element);
});
You can also select elements belonging to multiple classes:
var elements = document.getElementsByClassName("some_class another_class");
The main difference between the two is, querySelectorAll will allow you to select elements using CSS selectors, making it possible to select both by element name and by class name where getElementsByClassName only allows selecting by class name.
Tell us what you think:
Just a note , I dont see it mentioned but they return different types of collections. So there is a functional difference.
querySelector returns a static node list.
getElementsBy.. returns a dynamic html collection.
Also getElement is faster it uses a lot less back end parsing.
so generally if you can use it , use it because its faster, better supported(legacy) and is a dynamic rather than static collection.
Get Element By Id is a recursive method, and only looks from the global object. It's good for caching nested elements belonging to view in MVC pattern.
getElementById('main').querySelector('article');