Reading User Input in Bash
How to read user input from shell scripts using the read command.
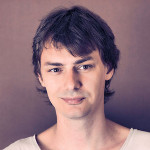
By. Jacob
Edited: 2023-08-18 15:01
#!/usr/bin/env bash
printf "\n Please type your username:\n\n"
read userName
if [ "$userName" = "" ]
then
printf "\nYou did not provide a user name, you ass clown!\n\n"
else
printf "\n\nHallo $userName""\n\n"
fi
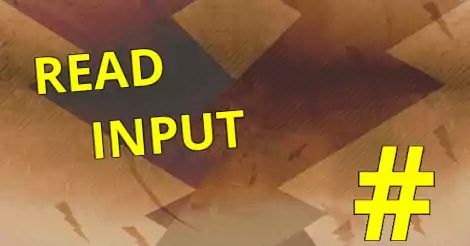
The above bash script is an example of how to await, read, and act upon the input provided by a user in a console environment.
1. #!/usr/bin/env bash — This first line is the shebang that defines the binary used to execute the script, in this case bash, and it should always be placed on the first line in a file. It would also work with other scripting languages, such as PHP and Python.
2. printf "\n Please type your username:\n\n" — This part simply asks the user a question by printing it. It is not strictly necessary, but it is good UX to include it.
3. read userName — This line makes the script "pause" while it is waiting for the users input. The input is stored in the userName variable.
4. if [ "$userName" = "" ] — This part is the if statement that makes a comparison of the input with something else. In this case we simply check if the input was empty, and admittedly it is not the best way to do so, but it shows you the basics of it.
Note. By including the -e option, we can even allow the user to use the TAB key to complete file paths, just like they would when using the terminal normally.
Accepting file system paths as input
When accepting file system paths as input, we should use read with the -e option, as this allows the user to use tab to complete file- and directory names.
read -e inputPath
However, this is not quite enough, since the user might also enter magic variables, such as the $USER variable, which will link to the users home directory. Simply replacing these special cases with the expected value is not always enough.
We also got the curious case of the tilde (~) character, which can be used as a "shortcut" to the users home directory. However, it can also be used to obtain the home directory of other users.
Because of this, we should take care to parse user supplied paths Properly.
See also: Using tilde in sh scripts
Convert user input string to lowercase
Here is a script from Project Humanize that will convert a user-supplied string to lower case. The script will ask the user for an input string, and then show the converted string in the console.
#!/usr/bin/env bash
printf "\n Supply the string to convert:\n\n"
read stringToConvert
if [ "$stringToConvert" = "" ]
then
printf "\nThe input was empty! Try again if you want.\n\n"
else
printf "\n\n "
echo "$stringToConvert" | awk '{print tolower($0)}'
printf "\n\n"
fi
Tell us what you think: