Generating Hashes From Bash and Terminal (SHA1, MD5 or SHA256. Etc.)
How to generate, and compare hashes from terminal using bash and PHP scripts in Linux.
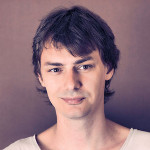
By. Jacob
Edited: 2019-09-30 03:09
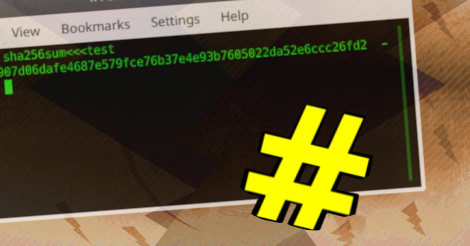
Sometimes I have had the need to generate a hash, be it MD5, SHA1 or sha256. In the past, I would do this from a PHP file, which i would then load via my browser. This is, however, hugely inefficient! So, I decided to look into how it could be done directly from a terminal in Linux.
This would appear not to be very obvious how to do, although I realized how do it later after much searching online.
In Linux, we have so-called "man" pages telling us how to use different commands. Using them takes some getting used to, as they are not very readable. However, they can still be helpful when trying to learn about commands used in bash scripting. To use it, simply type man followed by whatever command you want more information on:
man md5sum man echo
To generate a hash from terminal, one can use the hash functions:
md5sum<<<test
sha1sum<<<test
sha256sum<<<test
They also work for downloaded files, which can be useful when you need to verify a package has not been tempered with:
md5sum file-name.zip
If you are not currently in the Downloads folder, you can also type the path for the file, instead of first navigating to the ~/Downloads folder:
md5sum ~/Downloads/file-name.zip
This is all fine. But, what if you want to generate a hash from a CLI script? I do not really care what scripting language you use. You can use Bash if you are comfortable with that. Personally, I prefer the syntax in PHP scripts, but I am also learning to use Bash.
CLI Scripting
If you need to generate a hash from bash, things become more complicated. This is because extra characters might be added to your string, depending on circumstances. Both Bash and PHP scripts accept arguments. In PHP, they can be accessed via the $argv array:
#!/usr/bin/php
<?php
echo md5($argv[1])."\n";
The #!/usr/bin/php part tells the system to use the php binary to execute the script. The PHP scripts themselves can also be placed in /usr/bin, which makes them callable from anywhere. I would typically create a symbolic link for the original file, rather than having the script itself placed in /usr/bin.
The above can also be done in bash, and might look like this:
#!/bin/bash
echo -n $1 | md5sum
In bash, arguments are available in the $1, $2, $3, etc. Variables.
You could also ask the user a question, and then accept the string as input. But, since echo adds a newline character, the hash sum would be incorrect. The newline can be removed with -n (See: man echo in a terminal):
#!/bin/bash
# This script generates a md5sum from a string
echo "Type or Paste the String and hit enter to generate a md5 sum."
read inputString
echo -n $inputString | md5sum
The pipe character (Vertical Bar), can be used to take the output of one command (in this case echo), and serve it to another command. In this case, the first command getting executed is actually the echo command. The result from echo is then passed on to md5sum, and shown in the terminal.
You can test the above by excluding -n, which will result in a incorrect hash sum (the one with newline added).
Compare hash values with a bash script
Lets say you want to compare the hash sum of a downloaded file with hash'es found on the website, to verify the integrity of the download. Creating a bash script with a simple if statement would be enough.
#!/bin/bash
echo 'Supply your hash below (copy & paste):'
read inputHash
fileHash=($(md5sum $1))
if [ $inputHash = $fileHash ]
then
echo -e '\nMatched. The strings were:\n'
echo $inputHash
echo $fileHash
else
echo -e 'WARNING: NO MATCH!\n'
echo 'The file could be compromised. If you downloaded the file, you should try re-downloading it.'
fi
To use this script, simply do ./md5.sh some_file_name.iso
As you can see from the above, the syntax is not very nice, and takes some getting used to. The above can also be done with PHP, arguably in a more readable way.
Tell us what you think: