PHP: Arrays vs Objects
Learn about the difference between arrays and objects, and why objects are more powerful than arrays.
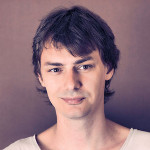
By. Jacob
Edited: 2023-10-14 21:51
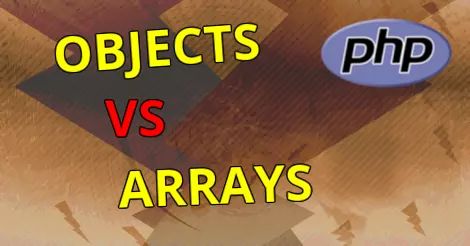
Objects can be a powerful way to create custom data structures to both contain and manipulate data. You can think about PHP objects much the same way you would think about physical objects in the real world; a car might have various features that allows it to drive, but at the same time it can also contain other things inside of it and be used for transportation of those things.
When it comes to choosing between associative arrays and objects for simple data storage and transportation, there is not really a clear answer as to what will be the "best", as it will often be down to personal preferences; however, objects will be more practical and powerful than mere arrays. Many developers will prefer objects because of the extra information they expose inside of their coding editor, but this information could just as well have been exposed with arrays. E.g. If you have an array definition somewhere, it should theoretically be able to expose the "known keys" to the developer, making it easier to access each key/value pair.
You can use an object to store key and value pairs in a similar way to how you would in arrays. When data is added to an object, it will typically be stored in properties (variables belonging to the object).
Typically a developer will often put restraints on how properties can be created and accessed, but if there are no such restraints, you could easily add new properties to objects by doing like this:
$myObject->newProperty = 'Hallo World';
echo $myObject->newProperty;
Ideally, restrictions put by the developer will prevent adding properties to objects, because doing so would modify the state of the object in potentially unsupported ways, which could, in worst case, break a system, or even compromise the security.
Why you should probably use objects
1. Objects is a more powerful and concise way to handle data than mere associative arrays, and the aspiring PHP developer will appreciate that objects may be used in similar ways as arrays. E.g. we may even use the foreach loop to iterate over the the properties in an object:
$containerObject = new stdClass();
$containerObject->one = 1;
$containerObject->two = 2;
foreach ($containerObject as $property_name => $value) {
echo $property_name .' '. $value . PHP_EOL;
}
Again, however, developer restraints might prevent us from doing that; an object might have defined its properties as "private", which means that we will not be able to access them directly. Instead the object might have a public method (a function belonging to the object), which we can use to access certain data within the object. E.g. An all_elements() method could return the results from a database query as an array, and we will often be able to loop over the output of such a function.
2. A great benefit of using objects is the fact that editors will expose public method- and property names when other developers are using our data structures, which reduce the risk of typos and other mistakes; if you were to use a non-existent method on an object you would get an error – with arrays the exact behaviour if you were to misspell an array key is more unpredictable, and potential resulting bugs can be harder to trace when using arrays.
3. Through the use of typed properties, you can define specific data types acceptable to a given property or method; if some other developer were to feed one of our methods an unsupported data type, they would get an error telling them exactly what they did wrong. In fact, you can even specify return types, so if an unexpected data type is suddenly returned, an exception will be thrown, and you will be able to more easily debug what has happened.
Anonymous classes in PHP 7
PHP 7 improved OOP even further by adding support for on-the-fly anonymous classes, avoiding the use of the generic, and clumsy, stdClass when spewing out object "data containers".
For example, the below example is created on-the-fly within an existing class:
// Anonymous class
$containerObject = new class() {
public $one;
public $two;
};
$containerObject->one = 1;
$containerObject->two = 2;
$containerObject->three = 3;
foreach ($containerObject as $property_name => $value) {
echo $property_name .' '. $value . PHP_EOL;
}
For all intends and purposes, this is the equivalent of creating a traditional class. We can even add magic methods to the class. For example, using __set() we may prevent adding properties from the outside:
public function __set($name, $value) {
throw new \Exception("Adding new properties is not allowed on this object");
}
Allowed characters in variables
As you may know, with arrays, we can use any string as a key name, but when using object properties we will normally be limited to using underscores, letters and numbers.
There is at least couple of ways around this problem:
- We can use variable variables to allow special characters in the variable name.
- We can use an array inside the object.
A dynamically named variable can be assigned like this:
$a = '##'; // Name of the variable
$$a = 'Hallo World'; // Data in the variable
echo ${'##'}; // Output the variable
Or, even easier:
${'%&¤'} = 'Hallo World';
echo ${'%&¤'};
To declare a property with special characters in an OO context, we may do like:
$myObject->${'##'} = 'Hallo World'; // Assign value
echo $myObject->${'##'}; // Output data
Tell us what you think: