PHP: Objects
Learn what PHP objects are, and how to use them in your own PHP coding adventures.
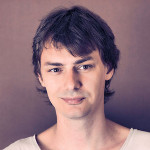
By. Jacob
Edited: 2020-07-27 10:59
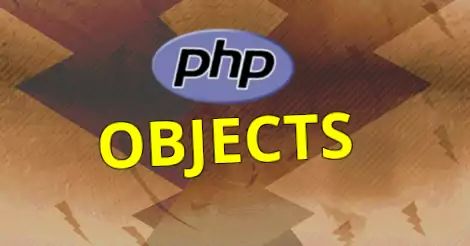
Objects are entities that are instantiated from their corresponding class definitions; in general, a well designed class should be loosely coupled with its dependencies, and not hide them from the authors who needs to use the class.
An object is really just the instantiated version of a class, and as such, the class definition itself might be more interesting to us than the instantiated object. When using type declarations we may also point to an object's class definition as the type; doing this will make a function or methods only accept an object of the given "class definition" as a parameter—which is a useful way to prevent authors from accidentally passing an incorrect dependency.
PHP class definitions
It is hard to discuss objects without looking at some code; so lets dive in and show an example of how to write a class definition, and then instantiate an object from the class.
In PHP a class looks like this:
class facts_about_the_world {
private $population_growth_rate, $shape, $base_year;
public $population_base;
public function __construct() {
$this->shape = 'Somewhat round anyway...';
// Note. The Population growth rate is not exponential, since it may vary from year to year
// Therefor, this can only be used to calculate a rough estimate..
$this->population_growth_rate = 1.0105; // https://ourworldindata.org/world-population-growth (2020)
$this->population_base = 7594000000; // (2020 estimate)
$this->base_year = 2020;
}
public function get_shape() {
return $this->population_base;
}
public function set_shape($value) {
$this->population_base = $value;
return true;
}
public function population_by_year(int $year) {
if ($year < $this->base_year) {
echo 'It is not possible to go backwards in time.';
exit();
}
$n = $year-$this->base_year;
// PHP uses "**" for exponentation instead of caret "^"
// See: https://beamtic.com/exponential-growth-php
return $this->population_base*$this->population_growth_rate**$n;
}
}
This is a very simple class that can show various facts about our world, and also estimate future world population in a given year.
To instantiate an object from this class, we may do like this:
$earth = new facts_about_the_world();
Then, in order to call public methods belonging to the class, we may do like this:
echo $earth->population_by_year(2050);
If a property is declared as public, we may also access it directly from the outside:
echo $earth->population_base;
But, directly accessing properties like this is usually not recommended, since it allows you to modify the properties as well; PHP still has no read-only keyword, so we will instead have to use getters and setters to manipulate object data safely.
Object Methods and Properties
The Methods (Aka. Functions) in this class are declared as public; the __construct method is called automatically when an object is instantiated from the class; this is also known as a magic method. It is also possible to define functions inside of methods, but this is not recommended.
Magic methods must be declared as public in order to work. It is also possible to declare methods as private, doing this has the effect that the method is only callable within the class itself.
Properties is a type of variable that is part of the class definition; it is also possible to use local variables inside of the individual methods; but, properties must be called with $this->property_name. The advantage of using properties is that they are accesible by all methods within a class without having to pass them on as parameters.
Getters and setters
Since declaring properties as public may have unwanted side-effects, we should instead declare them as private and then create getters and setters to help us access private properties.
PHP also has the magic methods __get and __set which are called when properties are accessed directly; but we may still want to create our own getters and setters, since it is more flexible.
The facts_about_the_world PHP class also has a private property called $shape, to access this property, we will have to use the get_shape method; now, this is a very basic method, since it only returns the value of the $shape property:
echo $earth->get_shape(); // Returns: Somewhat round anyway...
Now, to change the value of the private $shape property, we should use the method we specifically defined for this purpose:
echo $earth->set_shape('The Earth is completely flat...');
That is it for now, hope you learned what you wanted about PHP objects. Keep in mind this was just a beginners tutorial, there is still plenty of stuff to learn about when it comes to object orientated PHP.
Tell us what you think: