Foreach and Array_walk in PHP
How to use the PHP Foreach loop construct to iterate over arrays.
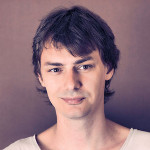
By. Jacob
Edited: 2021-02-21 15:02
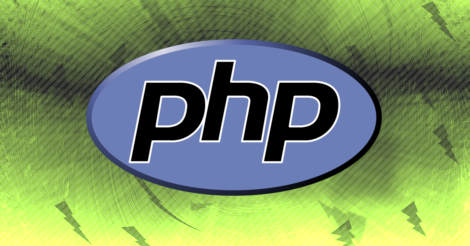
In PHP we have the foreach loop which is a developer-friendly loop construct that allows to iterate over arrays and objects.
The advantage of using a foreach loop instead of a while or for is that it is easier to use, since the array iteration happens automatically, without the need to think about counters or missing array keys.
$some_array = array('Apple', 'Banana', 'Orange', 'Carrot', 'Spinach');
foreach ($some_array as $key => $value) {
echo PHP_EOL . $key . ':' . $value;
}
Output:
0:Apple
1:Banana
2:Orange
3:Carrot
4:Spinach
As an alternative to foreach, you could also use array_walk with an anonymous function:
array_walk($some_array, function ($value, $key) {
echo PHP_EOL . $key . ':' . $value;
});
Using foreach with objects
The foreach loop works exactly the same way when using it to iterate over objects. When using objects, the object properties will take the place of "keys". It works well while strings and integers are used as values, but arrays and nested objects will need special treatment.
Here is an example of how to iterate over an object in PHP:
$containerObject = new stdClass();
$containerObject->one = 1;
$containerObject->two = 2;
foreach ($containerObject as $property_name => $value) {
echo $property_name .' '. $value . PHP_EOL;
}
exit();
The advantage of using objects instead of arrays for data storage is that objects are simply more powerful. You may even create your own class instead of the generaic stdClass.
The stdClass is just a generic empty class in PHP, it is useful for quick storage of data, but not so much for more advanced stuff.
If you are not sure what type the properties contain, you can use the "is_*" functions, here is an example using the is_string function:
foreach ($containerObject as $property_name => $value) {
if (!is_string($property_name)) {
break;
}
echo $property_name .' '. $value . PHP_EOL;
}
Tell us what you think: