Change Iframe src with JavaScript
Cool tutorial on how to change the iframe src attribute value using JavaScript.
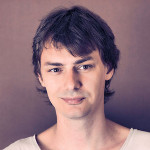
By. Jacob
Edited: 2021-02-09 21:27
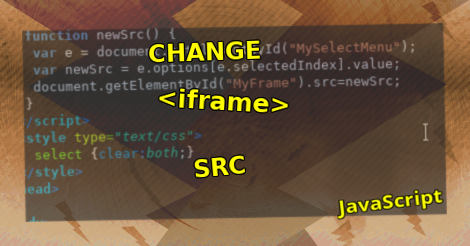
In this tutorial, you can learn how to dynamically change the src attribute of an HTML iframe using JavaScript.
Usually you would not want to use an iframe, unless you actually need to load external content; some sites also prevent "framing" of their pages, in which case you will probably need to download the content you want to display, and then serve it up from your local server.
If you just want to create a DHTML page that loads content dynamically, you may want to use the appropriate HTML sectioning elements and then load the content by using a combination of AJAX and innerHTML or DOM manipulation.
To change the iframe src attribute, we will be using a select drop down menu, and a button element to activate the function that will change the src attribute. Note that this is only for example purposes, instead of using onClick you may want to consider using event handlers in an external script.
The below is a fully working example. You can copy and paste it into a .html file on your computer, and open it in your browser. The JavaScript itself is explained in the subsections of this article.
<!DOCTYPE html>
<html>
<head>
<title>Change src value of iframe dynamically</title>
<script type="text/javascript">
function newSrc() {
var e = document.getElementById("MySelectMenu");
var newSrc = e.options[e.selectedIndex].value;
document.getElementById("MyFrame").src=newSrc;
}
</script>
<style type="text/css">
select {clear:both;}
</style>
</head>
<body>
<iframe src="https://beamtic.com/Examples/ip.php" style="width:450px;height:450px;overflow:scroll;" id="MyFrame"></iframe>
<select id="MySelectMenu">
<option value="https://beamtic.com/Examples/ip.php">Show IP</option>
<option value="https://beamtic.com/Examples/user-agent.php">Show User Agent</option>
</select>
<button onClick="newSrc();">Change Iframe Src</button>
</body>
</html>
Result:
Creating the Select Drop Down
The select menu consists of the select element, and multiple option elements, one for each site you want to allow in the iframe. I.e.
<select id="MySelectMenu">
<option value="http://yahoo.com/">Yahoo</option>
<option value="http://www.google.com/custom">Google</option>
<option value="https://beamtic.com/">Beamtic</option>
</select>
You should place this somewhere in the body of your HTML – it does not really matter where you place it, as long as its in the body.
You can now select the different options from the drop down, but before it will work, you will also need a means of getting the value of the selected option. For this we will be making a custom JavaScript function that can be called via the onClick event on a button. Therefor, you should create a button element somewhere. I.e.
<button onClick="newSrc();">Change Iframe Src</button>
The JavaScript Code
The custom function that we will be using is really simple, it only has 3 lines of code!
function newSrc() {
var e = document.getElementById("MySelectMenu");
var newSrc = e.options[e.selectedIndex].value;
document.getElementById("MyFrame").src=newSrc;
}
The first line will create a reference to the select element that we will be using, for simplicity we doing it by ID in this tutorial.
The second line fetches the value of the currently selected option element, which will then be handed over to the iframe in the final and third line of code.
How to block framing of your own content
To block iframing of your own content, you should add the x-frame-options: SAMEORIGIN response header; if you are using an Apache server, this can either be done in your host configuration, or in a seperate .htaccess file by adding the following:
header set x-frame-options SAMEORIGIN
Tell us what you think:
Hey Jacob, is it possible to dynamically change the iframe source leveraging the mainframe URL parameters as an ID and joining it with the URL in the iframe to render a detailed page? ex.
Mainframe URL: www.mysite.com?sfds654fsgfg6sfg54gfhghdf6
iframe src= "https://docs.google.com/spreadsheets/d/e/[google id to insert here dynamically from mainframe]/pubhtml?
Intended Final Result
iframe src= "https://docs.google.com/spreadsheets/d/e/sfds654fsgfg6sfg54gfhghdf6/pubhtml?
Hey Magnor, yes!
You would need to extract the sfds654fsgfg6sfg54gfhghdf6 parameter first. Also, don't forget about the trailing slash "/" at the end of the domain.
Usually you would have a value as well, so the parameter is not empty. I.e.: www.mysite.com/?sfds654fsgfg6sfg54gfhghdf6=something
In JavaScript, you can try
var my_parameter = location.search.split('sfds654fsgfg6sfg54gfhghdf6=')[1]
alert(my_parameter);
After this, it would be a simple matter of concatenating the two strings. Remember to wait for "DOMContentLoaded", otherwise you will just be accessing "myFrame" before it exists in the DOM. Full code:
document.addEventListener("DOMContentLoaded", function(){
var mainParameter = location.search.split('test=')[1];
var iframeSrcValue = document.getElementById("MyFrame").src;
var combined = iframeSrcValue + mainParameter;
alert(mainParameter);
});
Hi Jacob,
I'm trying to achieve almost exactly the same goal as Magnor Maxi did above, i.e dynamically change iframe src by website url.
I was able to get this far:
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML =
"https://dealtas.com/tracking"+window.location.search;
</script>
<body>
<iframe src="https://www.dealtas.com/tracking" width="100%" height="800px" frameborder="0"></iframe>
</body>
the script produces the full url I need but I don't know how to make that url be the iframe src
I don't have any knowledge in coding what so ever, thus would greatly appreciate any assistance.
thanks and keep safe
I'm trying to have the reply from a website embed in a page.
I don't even know how to ask the question, really.
I can send the payload, but it opens on the server site of the payload post. I'd like to open that reply in a frame on my page.
How?
Lane
How to change a youtube iframe embed url after user intraction on screen
ex : <iframe src="https://loadYoutubeEmbed/uI7fnrNhKSI" title="YouTube video player" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen="" width="560" height="315" frameborder="0"></iframe>
by on load in webpage and once user interacted on screen the iframe will change to src="https://www.youtube.com/embed/uI7fnrNhKSI"
its something like innerHTML.replace(new RegExp("loadYoutubeEmbed"),"www.youtube.com/embed")
any help available .
thanks in advance