How to Create a List with Selectable Items in an AutoIt GUI
How to create a list of selectable items with AutoIt.

Edited: 2020-07-27 20:32
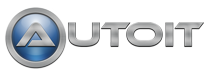
A common goal is to create a list of selectable items. In this AutoIt tutorial, we will create a list of three selectable items, a button to add more items to the list, and a button to return the currently selected item.
To create a list in AutoIt, we will be using the GUICtrlCreateListView function, we can then easily add more items to the list using the GUICtrlCreateListViewItem function.
When the list contains more items than there is room for, a scrollbar will automatically be added.
Creating the GUI with a list control
We will be using OnEvent mode in this tutorial, which is an easier way to handle GUI events. To enable OnEvent mode, we simply place the below somewhere at the top of the script.
Opt("GUIOnEventMode", 1)
This will toggle OnEvent mode on, and allow us to use the GUISetOnEvent function to bind events to our custom functions.
The below AutoIt script will create a simple GUI with a list containing three items, with just a single column. The _GUICtrlListView_SetColumnWidth function is used to set the width of the column, setting the width this way is much better than adding spaces to the column name.
#include <GUIConstantsEx.au3>
#include <GuiListView.au3>
Opt("GUIOnEventMode", 1)
MainGUI()
; ----- GUIs
Func MainGUI()
Global $listview
$listGUI = GUICreate("AutoIt list item GUI", 400, 200, 100, 200, -1)
GUISetOnEvent($GUI_EVENT_CLOSE, "On_Close_Main")
$listview = GUICtrlCreateListView("Users", 10, 10, 200, 150)
_GUICtrlListView_SetColumnWidth($listview, 0, 150)
GUICtrlCreateListViewItem("First Item", $listview)
GUICtrlCreateListViewItem("Second Item", $listview)
GUICtrlCreateListViewItem("Third Item", $listview)
$BtnAdd = GUICtrlCreateButton("Add Item", 10, 165, 80, 30)
GUICtrlSetOnEvent(-1, "Addi")
$BtnSelect = GUICtrlCreateButton("Select", 100, 165, 80, 30)
GUICtrlSetOnEvent(-1, "SelectItem")
GUISetState()
While 1
Sleep(10)
WEnd
EndFunc
; ///// Functions
Func Addi()
$sToAdd = InputBox("Add", "Enter Item Name", "")
GUICtrlCreateListViewItem($sToAdd, $listview)
EndFunc
Func SelectItem()
$sItem = GUICtrlRead(GUICtrlRead($listview))
$sItem = StringTrimRight($sItem, 1) ; Will remove the pipe "|" from the end of the string
MsgBox(0, "Selected Item", $sItem)
EndFunc
Func On_Close_Main()
Exit
EndFunc
When reading the list item, for some reason a pipe "|" character will be added to the end of the string, to remove this we simply remove the last character using the StringTrimRight function.
Tell us what you think: