How to Create GUIs in AutoIt
Tutorial on how to make GUIs using the AutoIt scripting language.
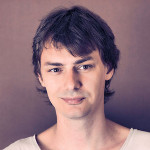
By. Jacob
Edited: 2019-09-11 16:21
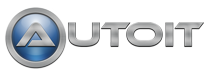
This tutorial shows how to create a simple GUI with two buttons, and how to make it perform a specific task depending on which of the buttons was clicked.
Before we start, it should be mentioned that there are more than one way to create GUIs. Which one suits your needs will be up to you to decide. In this tutorial, we will focus on just one way to do it.
You will find the full script at the end of the tutorial, we begin with the explanation of the script.
The first part of the script, located outside the main function, includes the GUIConstantsEx.au3 file, and sets the MustDeclareVars option, which makes it so that we must declare all our variables before using them.
#include <GUIConstantsEx.au3>
Opt('MustDeclareVars', 1)
MainGUI()
The GUIConstantsEx.au3 file included in the top of the script contains all the system events, such as the event of: maximizing, minimizing or closing a window.
The MustDeclareVars option makes it so that variables must be declared with either: Dim, Local or Global before use.
Finally the MainGUI custom function call just runs the MainGUI function, which you will find further down in the script.
The MustDeclareVars option makes it so we must declare variables before we can use them. Inside the MainGUI function, Local was used. Variables declared using local are only available at the local scope - in this case inside the function where they are declared.
Local $Button1, $Button2, $msg
The GUICreate function creates the GUI, assigns a title, and optionally controls the style of the GUI window. This function will be covered in a later Tutorial.
GUICreate("My GUI Window Title")
The GUICoordMode controls the coordinate mode to be used when placing new GUI elements, in this case the two buttons. I.e. Absolute or relative. This will also be covered in a later tutorial.
Opt("GUICoordMode", 2)
The GUICtrlCreateButton function is used to create buttons. This will also be covered in a later tutorial.
$Button1 = GUICtrlCreateButton("Button 1", 10, 30, 100)
$Button2 = GUICtrlCreateButton("Button 2", 0, -1)
In this example, the GUISetState function is used to show a newly created window.
GUISetState()
The while loop simply performs certain select statement checks continuously - in this example, the window only closes if $GUI_EVENT_CLOSE was performed.
While 1
$msg = GUIGetMsg()
Select
Case $msg = $GUI_EVENT_CLOSE
ExitLoop
Case $msg = $Button1
MsgBox(0, 'Button 1', 'Button 1 was pressed')
Case $msg = $Button2
MsgBox(0, 'Button 2', 'Button 2 was pressed')
EndSelect
WEnd
The full script
Finally, the full script. You can copy and paste this into a .au3 file, and try to run it.
#include <GUIConstantsEx.au3>
Opt('MustDeclareVars', 1)
MainGUI()
Func MainGUI()
Local $Button1, $Button2, $msg
GUICreate("My GUI Window Title")
Opt("GUICoordMode", 2)
$Button1 = GUICtrlCreateButton("Button 1", 10, 30, 100)
$Button2 = GUICtrlCreateButton("Button 2", 0, -1)
GUISetState()
; Run the GUI until the window is closed
While 1
$msg = GUIGetMsg()
Select
Case $msg = $GUI_EVENT_CLOSE
ExitLoop
Case $msg = $Button1
MsgBox(0, 'Button 1', 'Button 1 was pressed')
Case $msg = $Button2
MsgBox(0, 'Button 2', 'Button 2 was pressed')
EndSelect
WEnd
EndFunc
Links
- GUI Reference - autoitscript.com
Tell us what you think: