Disable and Enable Buttons in AutoIt GUIs
How to disable and enable AutoIt GUI elements using GUICtrlSetState.

Edited: 2017-03-25 02:42
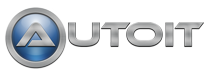
It can sometimes be useful to disable GUI elements when they are not used, or while the function they trigger is active – this is mostly to avoid that an action is performed more than required, but also to avoid confusion.
Users will often click a button multiple times if nothing appears to happen, even if the program is performing the requested operations and just doesn't clearly show it. Doesn't matter much with your private AutoIt scripts, but if you are going to distribute them, then you should try to avoid confusing the users of your programs.
This AutoIt tutorial will demonstrate how you can disable GUI elements, either until the function an element triggers has finished running, or until another button is pressed.
How to Disable and Enable Buttons in AutoIt
To disable any GUI element, we will be using the $GUI_DISABLE parameter of GUICtrlSetState – the below AutoIt script will disable and enable two buttons, depending on what button was pushed.
#include <GUIConstantsEx.au3> Opt('MustDeclareVars', 1) MainGUI() Func MainGUI() Local $Button1, $Button2, $msg GUICreate("My GUI Window Title") Opt("GUICoordMode", 2) $Button1 = GUICtrlCreateButton("Button 1", 10, 30, 100) $Button2 = GUICtrlCreateButton("Button 2", 0, -1) GUISetState() GUICtrlSetState($Button2, $GUI_DISABLE) ; Run the GUI until the window is closed While 1 $msg = GUIGetMsg() Select Case $msg = $GUI_EVENT_CLOSE ExitLoop Case $msg = $Button1 GUICtrlSetState($Button1, $GUI_DISABLE) GUICtrlSetState($Button2, $GUI_ENABLE) MsgBox(0, 'Button 1', 'Button 1 was pressed') Case $msg = $Button2 GUICtrlSetState($Button1, $GUI_ENABLE) GUICtrlSetState($Button2, $GUI_DISABLE) MsgBox(0, 'Button 2', 'Button 2 was pressed') EndSelect WEnd EndFunc
The important part is where we are using GUICtrlSetState, the select statement has already been explained in the first Tutorial. GUICtrlSetState takes two parameters, the first is the Control ID of the GUI element, in this case this is contained in the variables they where assigned to, $Button1 and $Button2 – we use $GUI_ENABLE when we want to re-enable a grayed-out gui element.
GUICtrlSetState($Button1, $GUI_DISABLE)
Disable Button While Actions are Being Performed
If you want to disable a button while some actions are being performed, then you need to use GUICtrlSetState in a similar way, how to do this is shown in the below example.
#include <GUIConstantsEx.au3> Opt('MustDeclareVars', 1) MainGUI() Func MainGUI() Local $Button1, $Button2, $msg GUICreate("My GUI Window Title") Opt("GUICoordMode", 2) $Button = GUICtrlCreateButton("Button 1", 10, 30, 100) GUISetState() GUICtrlSetState($Button2, $GUI_DISABLE) ; Run the GUI until the window is closed While 1 $msg = GUIGetMsg() Select Case $msg = $GUI_EVENT_CLOSE ExitLoop Case $msg = $Button GUICtrlSetState($Button, $GUI_DISABLE) ; disable the button ; Place your code or function call here GUICtrlSetState($Button, $GUI_ENABLE) ; re-enable the button when done EndSelect WEnd EndFunc
Tell us what you think: