How to Solve jQuery is Undefined Errors in Wordpress
There can be several different reasons why jQuery might be undefined; maybe it is not yet loaded, or maybe a script is unloading it. In this tutorial I will explain some of the problems and solutions that I have come by when developing Wordpress websites.
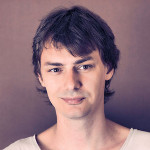
By. Jacob
Edited: 2021-02-13 15:49
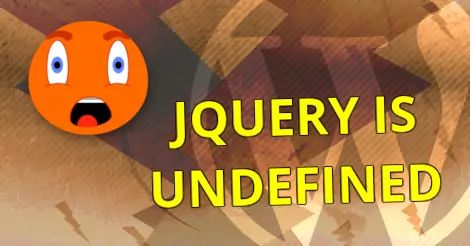
Wordpress has its own version of jQuery which is usually automatically loaded on each page request, and it is therefor recommended not to include your own downloaded copy. However, sometimes jQuery might not be loaded properly due to errors; in this article I will try to explain how to use jQuery within your Wordpress themes and Plugins, and how to debug some of the problems I have encountered.
It is important to note that Wordpress usually includes its own version of jQuery; including additional versions is only going to slow down your site, so if the one included by Wordpress does not work, you should really try to find out the reason rather than duplicating scripts.
A problem many beginners run into is that Wordpress will load jQuery as jQuery rather than $ — both of these are just variables that contain the instantiated jQuery object. Typically you will use jQuery like this in Wordpress:
jQuery(function () {
// Equivalent to the now deprecated jQuery.ready() or DOMContentLoaded
// Do stuff here
});
This is just plain beginners stuff, but it is still useful to mention, because a lot of beginners read my articles.
Sometimes jQuery might not be fully downloaded and loaded by the browser; in that case you might opt to use vanilla JavaScript to make sure jQuery is loaded before you try to use it. Since jQuery will normally be loaded when the page has finished loading, you can just listen for the DOMContentLoaded event before trying to use it:
document.addEventListener('DOMContentLoaded', function () {
jQuery("#example_box").text('Hallo World');
});
If jQuery or $ is undefined
If you are getting undefined errors, you should check if jQuery is being loaded properly. The easiest way to do that is to check that the script is requested by the browser in the network tab of developer tools.
If the script is being requested, it can be that another script is de-registering jQuery after it has loaded. To test for this, you can open developer tools from a browser like Chrome or FireFox and check the console.
Note. The shortcut keys to open developer tools is CTRL + SHIFT + i on your keyboard.
Once the page has loaded, try typing the following in the console:
console.log(jQuery);
If jQuery is loaded, then you should get a response like:
ƒ (e,t){return new S.fn.init(e,t)}
If jQuery is not loaded, you will get instead get a undefined response.
Wordpress will attempt to load jQuery in the jQuery variable, so, in order to access it, you will have to write your custom scripts like this:
jQuery(function () {
jQuery("#example_box").text('Hallo World');
});
However, you might still get jQuery is undefined errors depending on the sequence that your scripts are loaded in. Remember, jQuery must be fully loaded before you will be able to use it. A quick solution is to replace jQuery(function () {}); with a vanilla JavaScript DOMContentLoaded event listner:
document.addEventListener('DOMContentLoaded', function () {
jQuery("#example_box").text('Hallo World');
});
But, it would be better to change the location of the script. If you have placed the script in a template file, consider moving it to an external .js file that you can load via wp_enqueue_script.
Is jQuery unloaded by another script?
It could also be that one of your scripts is unloading jQuery. There is some sort of conflict handling for when multiple versions of jQuery are loaded, but it might cause problems when used incorrectly. The specific code might look like this:
let old_jQuery = jQuery.noConflict(true);
Note the noConflict(true) part; this is used when you want to use multiple versions of jQuery on the same page. The problem is that it will break scripts that relies on the global jQuery variable when used incorrectly. When used, the jQuery global variable might be set to undefined.
To find the code that causes this problem, you can open your website directory in an editor like Visual Studio Code, and then search the entire code-base for jQuery.noConflict — but do not just delete the occurrences, as that it may be unrelated and break things — instead you should look for occurrences in specific locations.
It is unlikely for Wordpress core, Plugin, and Theme files to contain errors, so you should mainly be examining your own code; this is usually restricted to code located in your child theme's folder, or inside the functions.php file, but you should also check in Code Snippets and other potential locations.
The offending code may also occur in the content of your website; to check if that is the case, you can search the database tables using phpMyAdmin or similar tools.
One of the downsides to Wordpress development is that you can change the code and functionality of a site in multiple places, which makes it hard to track down offending code when you got errors. I recommend making it your policy to only change things from central places, such as from within a child-theme.
Tell us what you think: