Files and Directories in AutoIt
Tutorial on how to read, write, and delete files with AutoIt.
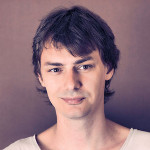
By. Jacob
Edited: 2019-09-11 16:18
AutoIt has build-in functions to perform read, write, and delete operations on files and directories.
While AutoIt can be used for much more than automation, a common task could be to clear temp files in Windows.
Writing data to a file
Before you either open a file for reading, or begin writing to a file, you should first use the FileOpen function to prepare the file for being handled. Optionally you may also want to check if the file was successfully opened.
$file = FileOpen("test.txt", 1)
If $file = -1 Then
MsgBox(0, "Error", "Unable to open file.")
Else
FileWrite($file, "Line1")
FileWrite($file, "Still Line1" & @CRLF) ; The CRLF at the end is a line break
FileWrite($file, "Line2")
FileClose($file)
EndIf
Sometimes a writing to a file might fail due to permissions, but this is not usually a problem.
Reading data in a file
In this script, reading a file is done using a combination of FileRead and FileOpen, and the data is being loaded into the $FileContent variable.
$file = FileOpen("test.txt", 0)
$FileContent = FileRead($file)
FileClose($file)
MsgBox(0, "Content:", $FileContent)
Deleting files
In AutoIt, there are a couple functions to delete files. FileRecycle will move files to the recycle bin, while FileDelete will delete them instantly.
To delete all files in a given directory using a relative path:
FileDelete("ScriptTemp\")
Using wildcards are also possible. For example, *.tmp will only delete files ending in .tmp
Wildcards such as *.tmp will only remove .tmp files, and not .tmping files. This is unlike the behavior of the FileFindFirstFile function, which will match both.
To move files to the recycle bin, and then empty it:
FileRecycle("MyDirectory\*.*")
FileRecycleEmpty()
To only empty the recycle bin for specific drives, use:
FileRecycleEmpty("c:\")
Create, and move directories
When you create a directory, you need to use the DirCreate function:
DirCreate("c:/New Folder/")
Moving a directory can be done with DirMove:
DirMove("C:\Source folder\", "C:\Parent\Destination Folder Name", 1)
The third parameter of the function is optional. It controls what happens if the destination folder already exists, in this case we explicitly set it to 1, which will overwrite the folder. The default setting is 0, not to overwrite.
Deleting directories
To remove a folder, you need to use DirRemove. The function takes 2 parameters, the first is the path of the folder that we want deleted, the second parameter controls whether sub-folders should be deleted as well. You will not be able to delete a folder that still has sub-folders, unless you set the flag.
DirRemove("C:\Parent\Destination Folder Name", 1)
Tell us what you think: