Solving: forEach is not a function
How to solve forEach is not a function in JavaScript, and information about why it happens.
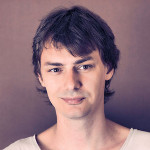
By. Jacob
Edited: 2020-07-12 15:41
If you are wondering why you get the message forEach is not a function, there is a very simple explanation for it; I have even had this happen on a data set that used to work in the past, which just added to my confusion.
The reason this happens is that you are trying to iterate over an object; and yes it might look like an associative array, but it is really an object.
There are multiple ways in which an indexed array might get turned into an object unintentionally; one way this can happen is if you somehow begin the creation of the index from "1" instead of "0" in your back end — at least that was what caused it when I was creating a JSON array from PHP.
There are two ways to solve it.
- The best solution is to make sure you got the correct data type to begin with; in order for the forEach method to work, you should be working with a JavaScript array.
- Another solution is to use the Object.entries() method.
let persons = {
a: 'Jacob',
b: 'Daniel'
};
for (let [key, name] of Object.entries(persons)) {
console.log(key + ': ' + name);
}
The above will create an object and iterate over it. If instead you wanted to use the forEach method, you should leave out the keys when building the data structure:
let persons = [
'Jacob',
'Daniel'
];
persons.forEach((name, key) => {
console.log(key + ': ' + name);
});
When JSON data is coming from PHP
It does not really matter where the data is coming from, but as an example let us say you using the json_encode function of PHP to create a JSON array for use in your front-end.
In my case, I was creating the array by incrementing a counter called $i; the name of the counter is irrelevant. What does matter, is the fact that I started the counter from "1" instead of "0"—and as many of you know, normally counters will begin at 0 in PHP.
This tiny detail caused the json_encode function to output a JSON object instead of a JSON array; and the problem with that is that forEach in JavaScript only works on arrays—there is no forEach method on objects!
Why it is a difficult problem to solve
Unless you are a JavaScript ninja, this behavior is unintuitive; perhaps especially for those with a PHP background.
The fact that JavaScript does not have associative arrays does not improve the situation, especially not when the syntax of JavaScript objects is very close to what a PHP developer would mistake as an associative array.
The PHP foreach loop will work on both objects and arrays in PHP. It would be great if that could work in JavaScript, as we would use a more straight forward syntax for iteration; this is perhaps even more important in JavaScript than in PHP, since JavaScript has no support for associative arrays.
Tell us what you think: