Hide or Show Error Messages in PHP
How to show or hide error messages in PHP. There are several ways to do this; from within the PHP scripts themselves, from php.ini, or from changing Apache configuration files.
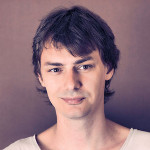
By. Jacob
Edited: 2021-02-07 23:08
There are a few ways to control error reporting for PHP scripts; often you would simply configure how to handle errors from within php.ini, but it is also possible to enable and disable errors from individual PHP scripts.
The error_reporting function controls the level of error reporting; you can either use a combination of values or a single value. Possible options include:
To disable errors from a PHP script, you may place the following somewhere towards the top:
ini_set('display_errors', 0);
ini_set('display_startup_errors', 0);
error_reporting(-1);
Errors should be disabled when using a custom error handler.
To enable errors, you may instead use the following:
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
When using a combination of values, each value should be separated by a pipe (|) character:
error_reporting(E_NOTICE | E_WARNING);
Finally, to only exclude a single error type, use the tilde (~) character in front of the type you want to exclude:
error_reporting(~E_WARNING);
Toggling errors in php.ini
How you choose to control errors is not usually important, but doing it from PHP is more friendly towards other developers, since they will more easily be able to make changes.
Sometimes it may make more sense to disable errors from within php.ini instead of attempting to toggle errors on/off from individual PHP scripts. It is recommended to disable errors in production environments, and only enable them doing development. Developers should have full control over their development environment, and therefor it is best to control error reporting from individual scripts or from php.ini directly — avoid using htaccess as much as possible.
Note. You can skip to step 2. if you already know where to find php.ini.
1. Errors can be toggled by adjusting the relevant settings in the php.ini file — the difficult part is to find the right .ini file to edit.
PHP will often have multiple versions installed; this possibly happens after updating, and might, for example, cause both PHP 7.3 and PHP 7.4 to be installed. The file you need to change is usually the latest version; but sometimes PHP-FPM might not load the correct version — that is a separate issue, and not something I will explain how to fix in this article.
But, how to approach it will mainly depend on how you installed PHP and which operating system you are using. For Debian and Ubuntu based Linux distributions, the php.ini file may be located in /etc/php/7.4/apache2/ if using the Apache module, or /etc/php/7.4/fpm/ if using PHP-FPM.
If you are having trouble finding the right file, you can create a script with the following content:
<?php
phpinfo();
Open the file in your browser and search for "php.ini" on the page. It should be located in the "Loaded Configuration File" row of the table.
2. Once you have located the file, you can change the following values to enable errors:
display_errors = on
display_startup_errors = on
error_reporting = E_ALL
Or, if you want to disable errors:
display_errors = off
display_startup_errors = off
error_reporting = -1
Using htaccess
If you are using the Apache HTTP server, you will also be able to toggle errors from .htaccess files. It is often recommended to disable htaccess in the server configuration in order to speed up the server, but this is probably not going to matter much for most people.
Toggling PHP errors from htaccess is done using php_flag in front of the php.ini option you want to change.
To turn on errors in htaccess:
php_flag display_startup_errors on
php_flag display_errors on
And to turn off errors in htaccess:
php_flag display_startup_errors off
php_flag display_errors off
The error reporting level is not easily controlled with htaccess.
Tell us what you think: