Submitting HTML Forms in JavaScript
To handle forms with JavaScript we may attach the preventDefault method to the submit event of the form, this allows us to handle the form data from our script instead.
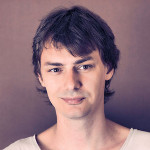
By. Jacob
Edited: 2020-03-12 01:55
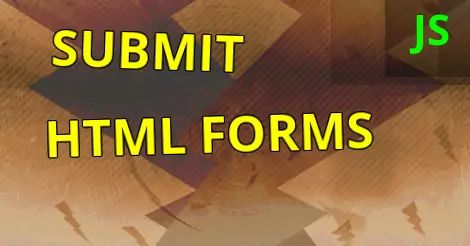
HTML Forms can be handled in JavaScript by preventing the default behavior of a form when it is submitted. However, by doing so we also prevent the form from submitting, and we will therefor often want to submit it later in the script. This can be done either by calling the submit method on the form, or by sending an AJAX request from JavaScript and silently send a HTTP POST request in the background, without changing the web page URL.
In many cases, it may make sense to both allow submitting the form normally—in case JavaScript is disabled in the users browser—and also allow submitting the form via JavaScript.
AJAX stands for Asynchronous JavaScript and XML, and has traditionally been used to communicate with the web server back-end. Nowadays, it is used more loosely, since you can also use JSON and plain text—the XML part is really not required. Still, using a format such as JSON will be easier than sending a plain POST request, since it avoids the need to manually url-encode POST parameters from JavaScript before sending a HTTP POST request.
As mentioned, we can prevent a form from submitting with the preventDefault method, a simple way to do this is shown in the below example:
let exampleForm = document.querySelector('#unique_id_of_form');
exampleForm.addEventListener('submit', event => {
// Prevent the default behavior of the submit button
event.preventDefault();
// Handle the HTML form data here!
let inputName = document.querySelector('#unique_id_of_form_input_field').value;
// Test with console.log() or alert()
console.log(inputName);
// alert(inputName);
// After handling the form data, submit the form.
// If you need to validate the form data, this is a good place to do it!
exampleForm.submit();
});
Submitting a HTML form with JavaScript
We can either submit the HTML form with the submit method, or we can create a HTTP POST request to silently submit the form. The latter option has the advantage that it allows us to update the page content dynamically depending on the server response.
Note. The submit method will not invoke the submit event on the form, but will instead submit the form directly.
A HTML form can be submitted using the submit method:
function form_submit() {
let exampleForm = document.querySelector('#unique_id_of_form');
exampleForm.submit();
}
Sending a HTTP POST request with parameters
To send a HTTP POST request from JavaScript, we will need to use the XMLHttpRequest object. I already made a HTTP client class to make things easier, so we will just need to instantiate a new HTTP client object from it to send an AJAX request.
Note. Since we are sending an Asynchronous request, we will need to handle the response inside a "callback" function. For simplicity I named the callback function in the example "callback", but you may name it whatever you like.
The below example shows how to send a HTTP POST request with the client class mentioned before:
let postSTR = "name=Jacob&status=active";
let postData = encodeURI(postSTR);
httpClient = new http_client();
httpClient.post("https://beamtic.com/Examples/form-submission-tester.php", postData, callback);
function callback(response) {
// Do stuff here
// Test that we got a response from the server
console.log(response);
// alert(response);
}
To update the page with the result from the submitted form, we may use innerHTML to access the element on the page that should be updated:
function callback(response) {
// Do stuff here
document.querySelector("#unique_id_of_html_element").innerHTML = response;
}
See also: Creating new Elements in JavaScript and Adding Content
Note. The postSTR variable in the above script can be filled out with content that we fetch from HTML form elements. To fetch the form data, we can simply use querySelector and pull it from the value attribute on the relevant input elements.
Each POST parameter needs to be separated by an ampersand (&), and finally we also URI encode the string with encodeURI before sending the request.
If you are dealing with a textarea, you could use innerHTML to get the content of the textarea element.
A working example:
Pushing this button will send a HTTP POST to the form tester: HTML Form Tester
Tell us what you think: