If Statements in AutoIt
Tutorial on how to work with If, Then, Else and ElseIf in Autoit.
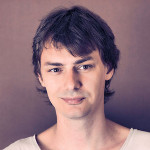
By. Jacob
Edited: 2019-09-11 16:22
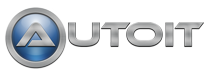
If statements are used to check if a given condition has been met, as in simple comparison. I.e. you can check if a counter variable has reached a certain count, and make your script do something depending on the result of the check.
A Single If
To perform a simple comparison, we will be using the If keyword to begin our statement, followed by the Then keyword, which controls what is to happen if the statement is true. I.e.
If $Var = 0 Then
MsgBox(4096,"", "The variable content was.")
EndIf
If you want to compare two strings, (I.e. text), you will have to use variables, as the syntax doesn't seem to support quotes.
$string = 'This is A test'
$string2 = 'This is A test'
If $string == $string2 Then
MsgBox(4096,"","Matched!")
EndIf
Note that we used two equals signs in the above example, this is called a operator, and the "==" operator is only used for string comparisons that you want to be case sensitive, simply use "=" if you don't want case sensitivity.
If Else
When you want something different to happen from what would happen if the statement returns true, you should use the Else keyword, followed by the EndIf keyword to finally end the statement.
$string = 'This is a test'
$string2 = 'This is not a test'
If $string == $string2 Then
MsgBox(4096,"","Matched!")
Else
MsgBox(4096,"", "The strings did not match!")
EndIf
If ElseIf
The AutoIt ElseIf gives the same result as a nested If statement, example below.
If $var > 0 Then
MsgBox(4096,"", "Value is positive.")
ElseIf $var < 0 Then
MsgBox(4096,"", "Value is negative.")
EndIf
Nested If Statements
It is also possible to include If Statements inside of other statements, an example of this is shown below.
$string = 'Some string 1'
$string2 = 'Some string 2'
$string3 = 'How to do If Statements'
$string4 = 'How to do If Statements'
If $string == $string2 Then
MsgBox(4096,"","Matched!")
Else
If $string == $string2 Then
MsgBox(4096,"", "The nested If was true")
EndIf
EndIf
Comparison operators
= | Check if two values match. Case insensitive when dealing with strings. |
== | Check if two values match. Case sensitive when dealing with strings. |
<> | Not Equal To. The comparison is case-insensitive when dealing with strings. |
< | Checks if the first value is less than the second. |
> | Checks if the first value is greater than the second. |
<= | Checks if the first value is less than, or equal to the second value. |
>= | Checks if the first value is greater than, or equal to the second value. |
Tell us what you think: