Fading HTML Elements With Jquery
In this tutorial you may learn how to create a fade effect using Jquery. With basic JavaScript it can be quite tough, and this is where Jquery may alleviate some of the coding burden.

Edited: 2019-09-11 16:32
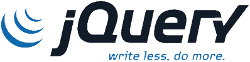
To add a fading effect to HTML elements using jquery is quite easy, we will be using the fadein and fadeout methods in this tutorial. We can either choose to use the strings fast or slow for the duration value, or we can supply the length of the animation that we want in milliseconds. I.e.
$('#fade').click(function() {
$('#item1').fadeIn('slow', function() {
// Animation complete
});
});
After including the jquery script file in our page, all we need to do is to place the above code in a script element in the body section of the page.
With jquery it is easy to extend an animation, so if we wanted the element to fadeout automatically, we would simply add some more code after the animation has been completed. I.e.
$('#fade').click(function() {
$('#item1').fadeIn('slow', function() {
// First Animation complete
$(this).fadeOut('slow', function() {
// Second Animation complete
});
});
});
Note the $(this) part used for the fadeOut function call, it can be used on subsequent calls to refer to the element being faded, that way you do not have to type the ID of the element over and over again.
Activating the fade when the page loads
Getting the fade effect to start when the page loads can sometimes be useful, and is a simple matter of using the .on method to attach an event handler, in this case we will use the load event handler, which will activate as soon as the element is loaded. I.e.
$(window).on("load", function() {
$('#item1').fadeIn('slow', function() {
// First Animation complete
$(this).fadeOut('slow', function() {
// Second Animation complete
});
});
});
In this example we attached the event handler to the window object, which will make the effect begin as soon as the page has been loaded. If you want to turn it on even earlier than that – before images, frames, and objects – use document instead of window.
Example
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>Using jquery to fade elements on a page</title>
<style type="text/css">
#item1 {
display: none;
}
</style>
<script src="jquery-1.10.2.min.js" type="text/javascript">
</script>
</head>
<body>
<button id="fade">Click here to fade</button>
<div id="item1">Just some textural content in a div...</div>
<script type="text/javascript">
$('#fade').click(function() {
$('#item1').fadeIn('slow', function() {
// First Animation complete
$(this).fadeOut('slow', function() {
// Second Animation complete
});
});
});
</script>
</body>
</html>
Tell us what you think: