Slide Effect with Jquery slideToggle
How to show and hide elements using the jquery sliding effects.

Edited: 2019-09-11 16:32
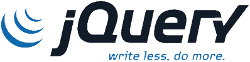
With jquery you can also create a sliding type of effect. Applied on a element that is initially hidden, you can make the element "slide down" to its full height – this can be a useful way to show more details to the users.
Ideally we want the element to slide down when we click a button, and hide again if the element is already visible – but first we'll show how to make the element become visible. The following script refers to a unique ID in the HTML source, the #slideButton ID, which is the ID of the button that will activate the jquery slide effect. I.e.
$('#slideButton').click(function() {
// Code here
});
The next thing we need to do, is to add the slide effect that we want to use, and select the element we want faded by its ID – in this case #item – We will be using the slideToggle function, which will automatically show and hide the element depending on its current state. I.e.
$('#item').slideToggle('slow', function() {
// Animation complete.
});
Which should result in the following JavaScript:
$('#slideButton').click(function() {
$('#item').slideToggle('slow', function() {
// Animation complete.
});
});
Hiding the element automatically after x seconds
In addition to the slideToogle function, we also got two other functions – the slideDown and slideUp. These functions can be used if you want more control over the behavior of the effects.
The slideDown will only work one time, and will not work if the element is already displayed, and the other way around with the slideUp – so this means if you want the element to hide through some other mechanism, you will have to code it yourself. For example, lets say you want the element to automatically hide after 4 seconds, you could use the JavaScript SetTimeout function. I.e.
$('#slideButton').click(function() {
$('#item1').slideDown('slow', function() {
setTimeout(function() {
$(this).slideUp('slow', function() {
// Animation complete.
});
}, 4000);
});
});
There are two things you should note here, the use of $(this) to refer to the last node, and the timeout in the second parameter of SetTimeout, defined in milliseconds.
Example
<!DOCTYPE html>
<html lang="en-US">
<head>
<title>Using jquery to show and hide elements with a slider effect</title>
<style type="text/css">
#item {
display: none;
height:200px;
border:1px solid #000;
}
#slideButton {
border:1px solid #000;
padding:0.3em;
}
</style>
<script src="jquery-1.10.2.min.js" type="text/javascript">
</script>
</head>
<body>
<div id="slideButton">Show More</div>
<div id="item">Just some textural content in a div...</div>
<script type="text/javascript">
$('#slideButton').click(function() {
$('#item').slideToggle('slow', function() {
// Animation complete.
});
});
</script>
</body>
</html>
Tell us what you think: