Add and Remove Classes with JavaScript and JQuery
You can either use a pure JavaScript solution, or you can choose to go with a library such as JQuery. Either way, this short tutorial show how it is done. Enjoy!

Edited: 2021-02-05 18:34
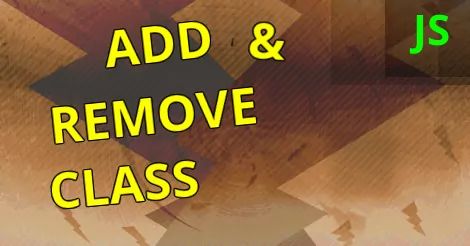
To add and remove a CSS class with JQuery is done with the addClass and removeClass methods.
Let us start by adding a class to an HTML element:
$("#unique_element_id").addClass("your_class_name");
If you later want to remove the class again, use the removeClass method like done below:
$("#unique_element_id").removeClass("your_class_name");
With pure JavaScript:
var e = document.getElementById("unique_id_of_element");
e.className += " name_of_class_to_be_added";
e.className = ""; /* Reset the class-list (remove classes) */
Adding or removing classes with pure JavaScript
While JQuery makes it possible to easily add and remove classes, it is almost equally easy with pure JavaScript. First you need to create a reference to the element, this can be done via the unique id of the element (defined with the id attribute). In JavaScript we may use document.getElementById() for this purpose:
var e = document.getElementById("unique_id_of_element");
e.className += " name_of_class_to_be_added";
To remove the class, you can simply set it back to its original state. That is, if there were any classes already present, you will need to list those. For example:
e.className = "some_class another_class";
When to use pure JavaScript instead of JQuery
The pure JavaScript way might be better in cases were you do not need the full functionality of a library. Sometimes you might want to avoid pure JavaScript solutions, as it can be difficult to make it work in all browsers. However, in this case it should be fairly safe. Try it out for yourself.
The only disadvantage of the pure JavaScript solution mentioned above, is that it requires space in it to separate from potential existing classes on the element. Beginners might find confusing, but it makes sense when you think about it.
There is also another way of doing it, but it is not supported in Internet Explorer 10. The browser is, however, very old (from 2012), so we should not support it. Tell users to switch to another browser, or upgrade their systems.
var element = document.getElementById("unique_id_of_element");
e.classList.add("new_class");
e.classList.remove("new_class");
Tell us what you think: