PHP: Sessions
Tutorial showing how to use Sessions in PHP.

Edited: 2018-01-14 05:43
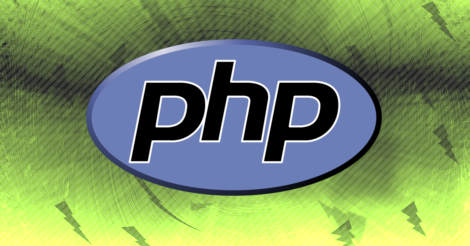
In PHP, Sessions are a useful way to store information temporarily. An example would be whether a user is logged-in or not.
PHP has build-in functions to manage sessions, which allow you to create login systems, and other fancy functions for your web applications.
How they Work
Sessions can be thought of as "variables". The values you store can be anything from e-mail addresses, login details (a hashed password, and a username). The values are accessible via the $_SESSION array, and are stored on the server-side, usually linked via a cookie on the users device. To create a session in PHP, you must first use session_start() before declaring any of the session values.
Below is the first page, lets call it index.php:
<?php session_start(); $_SESSION['UserName'] = 'Jacob'; ?> <a href="NextPage.php">Next Page</a>
The above will make PHP remember the name, "Jacob", in a session variable. The session is then linked via the cookie stored on the users device.
Note. Cookies are small text files which are stored locally on a users device. Cookies are typically used to remember when a user logs in.
The content of NextPage.php:
<?php session_start(); echo '<p>' . $_SESSION['UserName'] . '</p>'; ?>
Simply link normally from one page to the next, PHP automatically retrieves the session id in the cookie called "PHPSESSID".
Session IDs in URLs
It is also possible to store the session ID in the URL. Doing this will make the session work, even for people who have disabled cookies in their browser.
<a href="NextPage.php?<?php echo SID; ?>">Next Page</a>
There are two ways to link the session to the user. Either you need to use cookies, or you need to include the session id in the URLs. In general, it is recommended not to have session IDs in the URL, since it could cause problems when users are copying the URLs, and if search engines happen to index URLs containing session IDs.
Note. For people who have disabled cookies, it can be argued that it is their own responsibility to add your site to their trusted list in their browser. Cookies are dangerous, as often claimed in the media.
Destroying a Session
To destroy a session, or log the user out, session_destroy() may be used. However, you also need to clear the cookie with cookie(), like done below:
setcookie ("PHPSESSID", "", time()-60*60*24*100); // make the browser delete the cookie session_unset(); session_destroy();
Note. If your intention is to make a new session, you should instead use session_regenerate_id(). Only destroy the session when you need to logout the user.
Tell us what you think: