HTTP Headers are Case-Insensitive
HTTP headers are not case-sensitive, so we are free to convert them to all-lowercase in our applications.
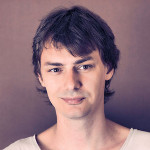
By. Jacob
Edited: 2020-08-18 16:11
When sending HTTP headers, we can safely normalize the header names into all-lowercase for consistency in our application; but the practical benefit is non-existent. While header names are case insensitive according to the HTTP specification, there is little reason to normalize the capitalization of header name — but doing so is safe.
If using PHP, we may use the mb_strtolower on each header name:
foreach ($headers as $key => $value) {
header(mb_strtolower($key, 'UTF-8') . ': ' . $value);
}
Why capitalization matters
I am not sure it is some kind of self-induced OCD that cause us to obsess about these tiny and insignificant things in our applications, but regardless of what it is, I will admit it — finding capital letters in my application headers is something that slightly annoys me.
Perhaps this happens because we spend so much time looking at tiny details — I am thinking of the kind where even a missing semi-colon or comma can stop an entire application from loading — but of course, this is not so much a problem using modern code editors such as Visual Studio Code, since code inspection usually alerts you of such problems; still, many developers use basic text-editors when coding, and I guess we might develop a type of sensitivity toward such inconsistencies. This is just pure speculation however.
I also think there are occasionally good reasons why developers prefer to normalize capitalization in various parts of our applications. One such place is in SQL queries where there is a long tradition to capitalize keywords in the statement. But, the problem with this practice is that some international users might struggle to read these capitalized SQL statements. When I learned this, I started writing all my SQL in all lowercase. The tradition only exists for readability reasons anyway; it is still helpful when writing SQL in a terminal where there is no syntax highlighting available.
Sometimes I wonder why we even got capital letters; some capital letters do not at all look the same in their capitalized version, and it is easy to understand why someone from another country would find this to be an inconvenience — we humans have so many ways to complicate things for ourselves.
Capitalization and HTTP headers
Since we rarely have to edit or look at HTTP headers, it really does not matter much that we got mixed capital letters with lower case letters in our header names.
I had a quick look at Google and Facebook, and they both seem to be sending lower case headers while Github sends the capitalized version. This means Github sends Content-Type: some-value while Google and Facebook sends content-type: some-value.
On the internet URLs are case-sensitive, which has lead wide-spread adoption of all-lowercase URLs; so it is perhaps not strange why some developers prefer same type of consistency in other areas of their applications.
Whether lowercase or capitalized header names are used also seems to depend on the server software. Keep in mind that we do not control all headers from PHP; some headers might be sent by the web server software. Apache, for example, is adding a server: Apache response header, nginx adds Server: nginx/1.7.1 , and varnish adds Server: Varnish
You can investigate headers more easily using cURL to send a head request, which will return just the headers:
curl --HEAD http://example.com/
Links
- 4.2 Message Headers - w3.org
Tell us what you think: