SQL: Select ... From
How to perform simple SELECT statements in SQL to communicate with SQL databases.
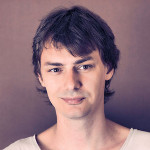
By. Jacob
Edited: 2021-02-05 23:42
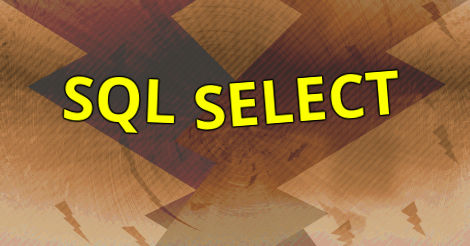
SQL A select ... from is performed either by choosing which fields you want returned or by using the asterisk (*) wildcard to return everything. Using the asterisk may be fine for testing and niche-situations, but only selecting the fields you actually need will often improve performance.
select * from table_name;
Using a WHERE clause allows us to only return specific results:
select * from users_table where email= 'peter@example.com';
Note. Examples uses lower case to increase the readability for international users, but you can use uppercase in order to highlight keywords. I.e.:
SELECT * FROM users_table WHERE email= 'peter@example.com';
Which to use is down to personal preference.
SQL select statements
To return a specific row, you can use the where clause. Depending on which field you use it on, you might end up selecting a single row or multiple rows. If you use where on a unique field, such as a username, then you should only get a single row.
A select is typically performed by deciding which fields you want returned from the database, this may look like this:
select username, password_hash, email from users_table where username = 'Jacob';
If this statement is entered in a command line, you might get a result like:
+----------+---------------+------------------+
| username | password_hash | email |
+----------+---------------+------------------+
| Jacob | ... | blah@example.com |
+----------+---------------+------------------+
1 row in set (0.00 sec)
You can also use asterisk (*) wildcard to select all fields in a database table, this is done like so:
select * from table_name;
Performing a wildcard select with a where clause:
select * from users_table where username = 'Jacob';
Optimizations
Avoid using the "*" Asterix in your SQL select queries to speed up your selects. Only select the fields you actually need.
Using indexes in your table will significantly speed up your selects.
Creating a combined index on columns that are commonly used in select combinations may be more efficient than creating individual indexes for each column.
Tell us what you think: