Remember to Add Comments to Your Code
Remember to write comments in your code to help future developers, and possibly even yourself.
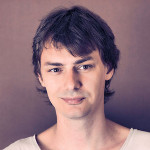
By. Jacob
Edited: 2020-10-26 18:47
Writing good comments is part of a documentation process when coding, and it should not be neglected when you are multiple people that work on the same code. Also, if suddenly you add a new team member that is less experienced with a CMS or framework, having comments in your code will help them learn their way around your system faster.
There are somewhat standardized ways to write comments, depending on the circumstances and language. If you are writing comments in PHP, you may benefit from studying the recommendations of https://www.php-fig.org/, and sometimes, also just reading other people's source code.
Once you are in a habit with writing comments in your code, it can actually be just as fun as the coding itself. Personally I always try to write comments in my code; my aim is to make my code as easy to use — and modify — as possible given the circumstances.
By circumstances I mean my own skill-level, and the design of my code or application. Like coding, writing good comments is something you learn with practice.
When to write comments
I am not religiously adding comments all over the place — some code is, of course, self-explanatory — but I probably do it more often than needed. Even if I just add some CSS to a Wordpress website's style.css file, I sometimes like to add a short comment to inform future developers what I have changed and why.
Most front-end HTML and CSS does not need comments when carefully coded. This is probably where I am a bit hard on newbies, but HTML and CSS is so simple to understand that it really should not require many comments. The only comments I add, usually, is to categorize my CSS declarations. Sometimes, when I got some CSS classes that are added or removed by JavaScript, I might add a comment informing the reader precisely what script is using the classes, and for what purpose. A CSS category comment might look like this:
/* Buttons on login page */
.dk_login_button1 {/* ... */}
.dk_login_button2 {/* ... */}
When I work on Wordpress sites, then I prefer to do things the right way from the start. This means I will either be making my own plugins, or I will be working on child themes. When working on Wordpress child themes, then it is more important to add comments about what you are changing; if you have copied an entire PHP class from the parent theme to the child (in order to override it), then it is useful to document exactly which parts in the class you are modifying, since it can be helpful if the theme is later updated and these changes needs to be re-implemented.
Always comment at the top of files
I always separate my PHP classes into individual files, and at the top of each file I like to write a short comment that explains the purpose of the class, as well as the author name. Such a comment might look like this:
/**
* Doorkeeper File Handler
*
* Class to handle creating, editing, and deleting files and directories with support for locking.
*
* write_file() should automatically handle locking, allowing use in some concurrency situations.
* This probably does not work for all applications, but should be enough in many cases.
* You may want to use a database for "heavy" load applications.
*
* @author Jacob (JacobSeated)
*/
Source: class: file handler
Avoid using multi-line comments in PHP files
Multi-line comments are best used at the top of files, or when you temporarily want to uncomment parts of the code — for everything else you should probably just use single line comments (//).
The reason for this is that it makes it easier to uncomment parts of the code.
The exception is when you write phpDoc blocks, these are comments at top of functions and class methods that helps document the code to your IDE; typically this allows a developer to hover a function name in an IDE to get information about how the function should be used. Again, an example from my own code:
/**
* A standard method to delete both directories and files,
* if the permissions allow it, a directory will be deleted, including subdirectories.
* @param string $file_or_dir Path to file or directory
* @return true
* @throws Exception on failure.
*/
public function simple_delete(string $file_or_dir) {
// ...
}
Source: class: file handler
Never add comments before the HTML doctype
Some older browsers will not play well if you add a comment — even a single white space character — before the doctype deceleration, and besides, it also looks ugly :-P This does not appear to effect modern browsers, but used to be a problem with old versions of Internet Explorer, causing it to revert to quirks mode.
<!-- This causes "quirks mode" in Internet Explorer -->
<!doctype html>
<html>
<head>
<title>test html page</title>
</head>
<body>
</body>
</html>
When a web page is rendered in quirks mode, it will not be rendered according to the web standards. This will cause different kinds of problems, such as inaccurate margin or padding on elements.
If you must add comments in this fashion to your HTML templates, then make sure they only exist in the source files, and not as part of the code on the live site.
<?php
// This comment is server-side, and causes no issue with browsers.
$title = 'some title';
?><!doctype html>
<html>
<head>
<title>echo $title; ?></title>
</head>
<body>
</body>
</html>
Links
- php-fig - php-fig.org
Tell us what you think: