PHP: Only Run Once at The Beginning or End of a Loop
How to most efficiently, only run a piece of code once at the beginning or end of a loop.
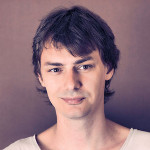
By. Jacob
Edited: 2022-11-16 07:41
I am not sure how to best title this problem, but I have often had situations where I needed to run a piece of code either first or last in a loop, and typically I would do stupid inefficient things like including an if statement inside of the loop. This should probably rarely be done; so what can we do instead?
The answer is surprisingly simple. If something needs to be executed first, do it right before the loop. If something needs to be executed last, do it after the loop.
Including an if statement inside a loop is inefficient when it only needs to be executed once.
This small piece of code will build a query string based on an array of parameters. The first part must always be a question mark "?", so you simply include it in the $query_string variable before starting the loop:
$query_string = '?';
foreach ($get_parms as $parm_name => $parm) {
$query_string .= $parm_name .'=' . $parm . '&';
}
$query_string = rtrim($query_string, "&");
I have been known to do crazy stuff like:
$query_string = '';
$first = true;
foreach ($get_parms as $parm_name => $parm) {
if ($first) {
$query_string .= '?';
}
$query_string .= '&' . $parm_name .'=' . $parm;
$first = false;
}
Or even worse:
$query_string = '';
$i = 0;
foreach ($get_parms as $parm_name => $parm) {
if ($i === 0) {
$query_string .= '?';
}
$query_string .= '&' . $parm_name .'=' . $parm;
++$i;
}
Only running a piece of code at the beginning or end of a loop is often needed when outputting HTML from PHP scripts. In my case, this would often be the case with HTML table, option lists, and elements like ol and ul.
If you, like me, have been confused about this, then you are not alone. I saw at least a few other people discussing a solution on stackoverflow; interestingly no one pointed out the most efficient and obvious solution.
What is the performance cost?
The performance cost of something like this is truly miniscule, but remember about Reasonable Efforts in Coding? Even tiny optimizations could matter on a high-traffic website; not to mention that some loops could be very long! With many concurrent requests, and multiple loops designed in this way, the speed benefit from doing it the optimal way might actually be noticeable.
Each time you increment a counter or assign a variable, it comes as a cost — you better avoid it entirely whenever possible.
Before realizing the best solution, I also considered calling a custom function that would call another sub-function, but function calls are said to be very expensive in PHP. It just shows, even something as "obvious" as this, can sometimes cause developers confusion.
Tell us what you think:
You are right. It's really confusing and I think there is no perfect solution for that. Especially, when you have "if" followed by "elseif" in the loop.