PSR: There must not be more than one property declared per statement
Why are we not allowed to have multiple properties in a single declaration?
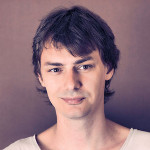
By. Jacob
Edited: 2020-12-20 13:24
There must not be more than one property declared per statement
I recently installed PHPCS with Visual Studio Code and enabled the PSR-12 standard. Looking to ways to improve my coding, I have had interested in the PSR coding standards for a while. However, I was disappointed to find that the PSR standard will also invalidate useful and harmless things, and I am currently not sure there is a way to turn it off.
This particular error apparently happens because someone has decided that you can not define multiple properties on a single line. This is a language feature, and one that I personally find very useful. I do not make perfect use of it, but same can be argued with the way people split up their functionality into multiple classes — there is no such thing as perfection when coding.
I personally enjoy lining up object properties separately from basic properties:
private $c, $db, $t, $sg, $th, $set;
private $site, $cookie_domain, $protocol, $visitor_ip, $request_path;
These two statements could even be shortened further, and separated by comments.
// Objects
private $c, $db, $t, $sg, $th, $set,
// Strings
$site, $cookie_domain, $protocol, $visitor_ip, $request_path;
Cleaning up, and getting things out of the way
So what is this about for me? Well mostly it is about saving vertical space in my code. while also organizing the code a little. I use the same technique when I write my CSS. It makes the code much more readable to me.
Presumably PSR will work best when multiple people work on the same code, to avoid too many inconsistencies when pushing code to GitHub. I can not really see how this should benefit the app functionality itself; it might even clutter the code somewhat when you are used to different ways of doing things.
The point of "one-line" statements is also to avoid code duplication. I am not sure it does anything performance wise, but it will help to clean our code; why someone would be against that makes no sense to me. You could even argue that PSR sould dictate the opposite, since having too many separate property declarations clutters the start of the class definitions. I rarely need to touch the properties once they have been declared, and so, prefer they just stay out of my way.
Underscores
If we look up the particular rule in the PSR12 standard, then we will also find the following rule:
Property names SHOULD NOT be prefixed with a single underscore to indicate protected or private visibility.
This actually does make sense to me, because the autocomplete feature in our programming tools will typically only suggest public properties when working outside the class. And, at least in Visual Studio Code with Intelephense, we can also hover function and method names to get a quick-reference on how it is supposed to be used.
This also brings me to my next point of criticism of the PSR12 standard. Apparently someone also decided that underscores are not allowed in class names, and we should instead use PascalCase which is harder_to_read; I do not mind people using pascal case, and I will never change an existing format if I am modifying other people's code, but I sure would not like to make rules about it. It makes no practical difference. You can even mix the style throughout your code — it does not matter.
To push this madness further, we are also not allowed to use underscores in property names, instead we should use camel caps:
private function whatYouSay() {
// ...
}
Hell, someone even decided that periods "." should be surrounded by at least a single space when concatenating strings. Of course, this may be of some use when working multiple people on same code, to avoid too many code changes when pushing to git. Nevertheless, I sometimes wonder if some developers suffer from a severe case of OCD, and just needs everything to be done in certain ways in order to be able to focus on the code itself.
Links
- Properties and Constants - php-fig.org
Tell us what you think: