See if a Checkbox is Checked in PHP
Short totorial on how to work with checkboxes in HTML and PHP.

Edited: 2020-03-03 23:42
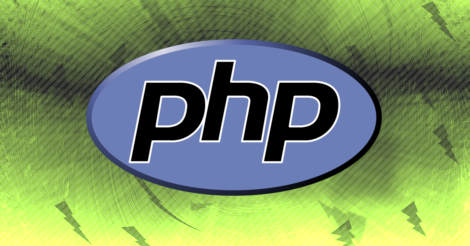
Checkboxes can be used in HTML forms to control settings related to whatever is being posted back to the server. For example, if you are creating a new blog post from a HTML form, checkboxes can be used to control whether certain data should be hidden or shown to your visitors when viewing the blog post.
If a checkbox is checked, the value will be included in the post. Therefor, keeping a static value of 1 should be sufficient in most cases. The name attribute allows you to distinguish the box from other form elements, so the value is not really that important.
To check a checkbox you can use the checked attribute, or alternatively avoid including it to leave the checkbox unchecked.
In your HTML, a checkbox should look like these below (all valid examples).
<input type="checkbox" name="mycheckbox" value="1" checked="checked">
<input type="checkbox" name="mycheckbox" value="1" checked>
Styling and placement of form elements is not part of this tutorial.
Note. Extra care should be taken to make sure your HTML form elements are accessible.
Form labels should have a for attribute that correspond with the ID of the input element that they are associated with.
Checking if a checkbox was checked
If a checkbox is checked, the value will be included in the HTTP POST and made available in the $_POST superglobal array. So, to find out if a box was checked, we can use an if statement to see if mycheckbox is found in the $_POST array. You can either use empty, or isset. It does not really matter, as long as you understand behavioral differences.
if (empty($_POST['mycheckbox'])) {
echo "Checked!";
} else {
echo 'Not checked!';
}
The above looks rather messy if you have a lot of code, so you may want to shorten the if statement, and keep it down to one line. The below will set the $my_checkbox variable to 1 if your checkbox is checked, or an empty string if not checked.
$my_checkbox = (empty($_POST['mycheckbox'])) ? 1 : '';
Since we are not using the value from our checkbox, the $my_checkbox variable can safely be inserted directly into a database without validation.
Tell us what you think: