Handling HTML Forms in PHP
A tutorial that shows how to handle HTML form submissions from PHP scripts.
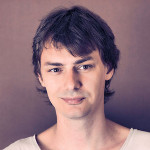
By. Jacob
Edited: 2020-03-03 23:33
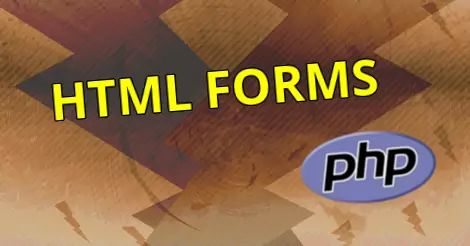
Whether subscribing to a e-mail newsletter or submitting a contact form, the data in the HTML form will typically be sent to a server sided script to be worked with further.
With PHP, we can easily handle form submissions via the $_POST and $_GET superglobal variables.
To return the value of a specific input field, simply use the value of the field's name attribute:
if (!empty($_POST['e-mail'])) {
echo $_POST['e-mail'];
}
If the names of the form fields are unknown, then we can learn them either by printing the relevant superglobal, or by iterating through it using a foreach loop:
echo '<p>The submitted data were:</p>';
foreach($_POST as $key => $value) {
echo 'Key: <b>'. $key . '</b><br> Value: <b>' . $value . '</b><br><br>';
}
echo '</pre>';
A test-form is available here: HTML Form Tester
Also do not forget about our old friend, print_r:
print_r($_POST);
Note. Same approach can be used with the $_GET superglobal.
However, it is one thing to throw a ball and catch it, and another to also score a goal with the ball after catching it. Meaning that, often it can be difficult to do something useful with the data after "catching" it with our server-sided PHP script.
We can easily show the data to a user in a web browser, and often this is all we want while developing the submission handler. But, sending a message via e-mail requires more work. In many cases, our web-host will already have prepared some things we need, but in other cases we might need to setup our own e-mail server in order to send e-mails from PHP.
The below script will "catch" the data submitted by any form, and output it to a web-browser. This is useful when developing new form-submission handlers, and learning about HTML form handling in PHP:
Catching submitted form data from PHP
How to handle the submitted data from PHP will depend on the type of form input field we are dealing with. Text fields will always be included, even when they are empty, this makes them easy to deal with. Checkboxes and Radio Buttons will need special treatment, since they are only included if they have been checked.
However, even checkboxes and radio buttons can be easily handled—you just need to remember that they will only be included if checked. Knowing this, we can use isset to test if a given checkbox or radio button was selected.
We can check that a normal form-field was filled out using the empty function, but you should remember to also validate the content to prevent errors and injection-attacks. To check if a text field was filled out, use the below:
if(!empty($_POST['some_field_name'])) {
echo 'Error: You need to fill out all required fields in the form!';
exit();
}
// The rest of your code
echo 'Value of form field: ' . $_POST['some_field_name'];
Note. The key value used in the $_POST or $_GET superglobal is contained in the HTML name attribute of the form element.
Selectboxes and Radio buttons
For HTML radio buttons and checkboxes, the contents of the value attribute rarely matters, and we can simply check if the box was checked by the user using isset:
if (isset($_POST['contact_me'])) {
echo 'The contact_me checkbox was checked!';
} else {
// Do something else
}
// The rest of your code
Multiple Radio buttons can have share the same name attribute—but the ID should still be kept unique.
Since only the value of the radio button that was checked will be submitted, this allows us to check which radio button (option) was picked by looking at the content of the value attribute:
if (isset($_POST['selected_option'])) {
echo 'You selected: ' . $_POST['selected_option'];
}
Tell us what you think: