Using proxy servers with cURL in PHP
Tutorial on how to use proxy servers with cURL and PHP
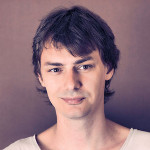
By. Jacob
Edited: 2023-05-16 06:45
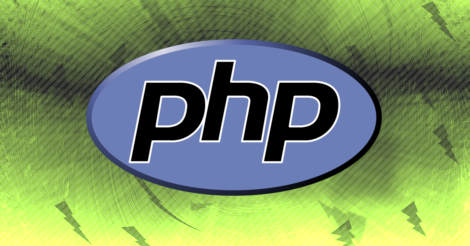
Setting a proxy server to be used with cURL and PHP is relatively simple, it mostly depends on the server that you are using, and authentication method (if any). The HTTP authentication method is controlled with the CURLOPT_PROXYAUTH option, the default method is CURLAUTH_BASIC – if the proxy requires authentication, a username and password can be set in the [username]:[password] format, using the CURLOPT_PROXYUSERPWD option.
Note. instead of using proxy servers, it can be better to use a VPN connection inside a virtual machine or a Docker container, because it will be separated from your main OS, and the connection might be faster and more stable.
Using public proxy servers is not recommended, and it might even be illegal – you should examine this carefully before using any proxy servers that you might find. In addition, proxy servers – perhaps especially public ones – could be under the control of hackers that are using them to spy on the traffic going through the server. You should be very careful about using public proxy servers for these reasons.
For now we'll just focus on using a proxy that doesn't require any authentication. Setting a proxy server and a port number in PHP for cURL can be done using the CURLOPT_PROXY option, like shown in the below example:
curl_setopt($ch, CURLOPT_PROXY, '128.0.0.3:8080');
As shown in the above example, you can set the a proxy with the IP:PORT syntax in PHP using cURL. But if you prefer to keep the ip seperated from the port, you can also use the CURLOPT_PROXYPORT option, which would result in the below PHP code:
curl_setopt($ch, CURLOPT_PROXY, '128.0.0.3');
curl_setopt($ch, CURLOPT_PROXYPORT, '8080');
After setting a proxy server, you will be able to perform the request using the curl_exec function. I.e.
$ch = curl_init($url);
$url = "http://beamtic.com/Examples/http-post.php";
curl_setopt($ch, CURLOPT_PROXY, '128.0.0.3');
curl_setopt($ch, CURLOPT_PROXYPORT, '8080');
// Perform the request, and save content to $result
$result = curl_exec($ch);
echo $result;
Setting cURL Proxy Type
cURL supports two proxy types, the default is HTTP, and the other option is SOCKS5. You can set the proxy type using the CURLOPT_PROXYTYPE option. I.e.
curl_setopt($ch, CURLOPT_PROXYTYPE, CURLPROXY_SOCKS5);
You really only need to set the type of the proxy, if you are not using a HTTP proxy.
Setting Authentication Method
As mentioned in the beginning of the tutorial, setting the authentication method of a proxy server can be done using the CURLOPT_HTTPAUTH option. To make this work properly, we will also need to provide a username and password for the proxy server, this is all accomplished in the below script, in which we are just using a BASIC authentication method.
$ch = curl_init($url);
$url = "http://beamtic.com/Examples/http-post.php";
curl_setopt($ch, CURLOPT_PROXY, '128.0.0.3');
curl_setopt($ch, CURLOPT_PROXYPORT, '8080');
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
// The username and password
curl_setopt($ch, CURLOPT_PROXYUSERPWD, 'USERNAME:PASSWORD');
// Perform the request, and save content to $result
$result = curl_exec($ch);
echo $result;
Other authentication methods include the following:
- CURLAUTH_BASIC
- CURLAUTH_DIGEST
- CURLAUTH_GSSNEGOTIATE
- CURLAUTH_NTLM
- CURLAUTH_ANY
- CURLAUTH_ANYSAFE
Note.The vertical bar | (or) operator can be used to combine methods. If this is done, cURL will poll the server to see what methods it supports and pick the best.
Tools:
You can use the following API endpoints for testing purposes:
https://beamtic.com/api/user-agent
https://beamtic.com/api/request-headers
Tell us what you think: