PHP Data Types
This tutorial is just a short beginners introduction to data types in PHP.
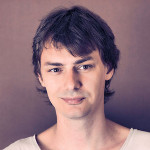
By. Jacob
Edited: 2022-04-03 10:16
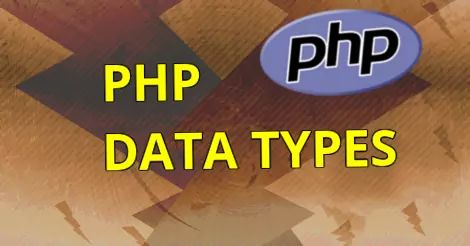
PHP does not support explicit data type definitions for variables, and instead, the different types are determined by the context in which variables are used. Often, PHP will automatically convert data types as needed.
However, PHP still has a number of different data types which are useful to know about when doing validation and comparison of variables.
It is still possible to assign different data types to variables, and implement strict type validation. You may already know about the string and integer types, but an array is also a data type, and we can use PHP's build in function to check for different types.
For example, if you want a variable to contain a integer data type, you should assign the variable without using quotes:
$some_variable = 500;
While a string would be assigned like this:
$hallo_str = 'Hallo World';
We could then make sure the variables contain the expected type by using is_int or is_string:
if (is_string($hallo_str)) {
echo 'The variable was a string.';
} else {
echo 'The variable was not a string!';
}
Numbers and strings
A number may be kept as integer, float, and text may be stored as the string data type.
Some also consider it good coding practice to append the data type to the name of the variable—note the _int at the end:
$some_variable_int = 500;
$hallo_str = 'Hallo World';
Numbers can also be assigned as a string data type, the consequence is that certain numeric operations might fail, so it is generally always advisable to us a integer type for whole numbers.
Since integer literally only covers whole numbers, you will need to use a float data type for decimal numbers. You can assign a float just like you would assign an int:
$some_variable_float = 100.0;
var_dump($some_variable_float);
Output:
float(100)
The difficult part is to compare and validate different data types without breaking things. For example, if you have programmed your application to accept a int value, and it suddenly receives a float instead, you will just have introduced a bug in your code.
Sometimes, it is best just to leave the type juggling to PHP, since a variable's type is determined by the data assigned to it. This means, in some cases, rather than validating a variables type with a function such as is_int, you would instead trust that the content of the variable is valid. Alternatively, you should make sure that all valid types are checked for. I.e:
my_currency_function($amount) {
if ((!is_int($amount)) || (!is_float($amount)) ) {
echo 'Invalid input type.';
exit();
}
// If the type was valid we continue....
}
List of data types in PHP
The following is a list of data types in PHP 7.
Scalar types:
- string
- integer
- float
- boolean
Compound types:
- object
- array
- callable
- iterable
Special types:
- resource
- NULL
Tell us what you think: