PHP String Interpolation, Using Variables in Strings
How to effectively use variables within strings to insert bits of data where needed.
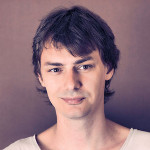
By. Jacob
Edited: 2021-12-07 01:23
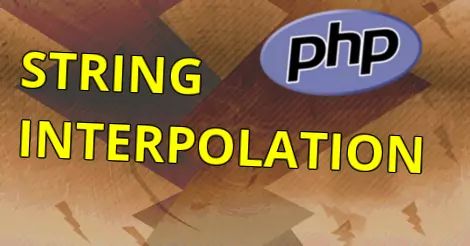
String interpolation is the process of replacing variables in a string with the content of the variables. This variable interpolation is made possible when the string is either enclosed in double quotes, or when a heredoc is used.
Variables can either be included in the string using a simple syntax, or by surrounding the variables with curly brackets, also known as complex syntax. Using curly brackets allows us to explicitly specify the end of the variable name, which would otherwise risk getting mixed up with the string.
Beginning with the simple syntax:
$some_more_text = 'and some more text';
$my_variable = "This variable contains some text, $some_more_text";
// Output the interpolated variable
echo $my_variable;
Note. Only use Double Quotes if the string either contains variables or escape sequences that needs to be interpreted.
The above syntax will not work when we got text surrounding the variable name itself. To solve this, we should use complex curly syntax:
$alphabet_fragment = ', h, i, j, k';
$alphabet = "a, b, c, d, e, f, g{$alphabet_fragment}, l, m, n...";
echo $alphabet;
Double Quotes for Interpolation
Generally, it is bad to use the simple syntax for interpolation, since you can easily break the interpolation by accident, and it can also be bad for the readability of your code.
There is a couple of things you can do to increase consistency, and avoid breaking the interpolation:
- Use String Concatenation to combine variables with your strings.
- Use Curly Brackets syntax for interpolation.
Personally, I prefer to use concatenation for interpolation on small strings, and Curly Brackets when using heredocs for loading template files or working with larger strings.
Using concatenation:
$alphabet_fragment = ', h, i, j, k';
$alphabet = 'a, b, c, d, e, f, g' . $alphabet_fragment . ', l, m, n...';
Using curly brackets with heredocs
When you are using the heredoc syntax, you will most commonly be working with large strings. One scenario where this is useful, is when keeping a HTML template in a PHP variable.
However, heredocs also increases the chance of variable-text mixups, which makes the complex syntax particularly useful in heredocs:
$template = <<<_LOADTEMPLATE
<p>{$some_variable['hallo_world']}</p>
_LOADTEMPLATE;
// End of the heredoc
Tell us what you think: