How to Create Wordpress Plugins
Beginners tutorial on Wordpress Plugin development using an objected orientated approach.
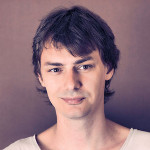
By. Jacob
Edited: 2020-07-26 15:24
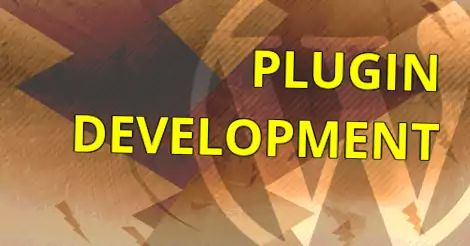
Creating plugins for Wordpress is fairly straight forward, and makes it possible to modify and add new features to existing Wordpress installations that are otherwise difficult to maintain and implement.
Of course, there is already a very large library of plugins that are available for free online, and chances are that there already is a plugin for what you want to do. However, these plugins may come with annoying ads to "opgrade" or buy a "pro" version of the plugin; or worse, they might come with security vulnerabilities. These are reasons enough to want to create your own plugins.
Creating Wordpress Plugins is also better than messing with the functions.php file. I have created a few plugins over the years, and more recently I found work that required me to make plugins for Wordpress.
Creating your first plugin
Wordpress plugins are located in: /wp-content/plugins/, to create a new plugin, all we have to do is to create a new directory in this folder, and then place the files belonging to our plugin within this new folder. The exact steps are covered below.
1. Create a new directory at /wp-content/plugins/name-of-new-plugin/
2. Create the plugin "index" file (Aka. the composition root of the new plugin) /wp-content/plugins/name-of-new-plugin/name-of-new-plugin.php
3. Now, we may edit the composition root of our plugin, that would be the name-of-new-plugin.php file located in /wp-content/plugins/name-of-new-plugin/.
To safely test the new Plugin, we can write the following in the file:
<?php
/**
* Plugin Name: Name of New Plugin
* Plugin URI: https://example.com/
* Description: Plugin to test things
* Version: 1.0
* Author: Beamtic
* Author URI: https://beamtic.com/
*/
// My first Wordpress Plugin :D
if (false === isset($_GET['plugin_test']) || 'true' !== $_GET['plugin_test']) {
return false;
}
echo phpinfo();
exit();
I should not have to explain basic PHP in this tutorial, but, nevertheless, the $_GET['plugin_test'] part will check if the plugin_test URL parameter was used; this avoids executing the plugin code on every page request until we are ready to do so. If the parameter is not found, we simply return false without executing the plugin code at all.
Since plugins are loaded before output is sent back to the client web browser, this above code would output the phpinfo content and then immediately exit. The phpinfo function can be used understand your environment better, and avoid using features not supported on your server.
4. Go to Plugins in your site's controlpanel, and enable your new plugin.
5. In order to safely test the new plugin, the URL parameter ?plugin_test=true is used—this is a very useful way to test new plugins on a live server without having them effect normal operation of the site.
To test the plugin, after activating it, you just have to add the ?plugin_test=true to an URL on your domain name. I.e:
https://example.com/?plugin_test=true
// Or
https://example.com/some-page/?plugin_test=true
Note. Adding new plugins may cause a blank page if you are not careful doing development. To avoid this, using a test parameter, as recommended in this tutorial, will avoid locking yourself out of your Wordpress site due to fetal errors caused by errors in your plugin.
Wordpress plugin development
There are two ways to develop wordpress plugins; one is to use an object orientated approach, and the other is to use basic procedural code. In these tutorials, I will be focusing on the object orientated approach, since it increases the portability and maintainability of your code; I will however also show how to easily convert procedural code to OOP.
We have not done anything useful with the new plugin yet. So, let us try to add some content to the page; one thing to keep in mind is that Wordpress has its own ways to add JavaScript and CSS code, I will get to that later in the tutorial—so do not add style script elements directly in the HTML content out of desperation.
In Wordpress development, we will need to use so-called "hooks" to hook-into (engage with) the parts of the content that we need, and "filters" to manipulate the content. For example, there is a hook for the main page content, and there is a hook for footer content. Let us try to add some text to the main content.
Adding content from Wordpress plugins
To add content to pages, we should use the hook called the_content.
In order to do this the Procedural way, we can do like this:
if (false === isset($_GET['plugin_test']) || 'true' !== $_GET['plugin_test']) {
return false;
}
add_filter('the_content', 'my_content_filter');
function my_content_filter($wp_content)
{
$my_content = '<p>Hallo World!</p>';
// Add our content before the existing content
$new_content = $my_content . $wp_content;
return $new_content;
}
Procedural code might be useful to test smaller things, so I suggest you setup a "test" or "playground" type of plugin, where you can just test off things before implementing them in your other plugins.
This, when the plugin is enabled, and you have used the "plugin_test" parameter, should output:
<p>Hallo World!</p>
At the top of your content.
Using Object Orientated PHP
To convert the above procedural code to Object Orientated, all we have to do is to create a PHP class to contain the code. The final file should look like this:
/**
* Plugin Name: Name of New Plugin
* Plugin URI: https://example.com/
* Description: Plugin to test things
* Version: 1.0
* Author: Beamtic
* Author URI: https://beamtic.com/
*/
if (false === isset($_GET['plugin_test']) || 'true' !== $_GET['plugin_test']) {
return false;
}
// Create object from class
$name_of_plugin = new name_of_new_plugin();
class name_of_new_plugin
{
function __construct() {
add_filter('the_content', array($this, 'my_content_filter'));
}
function my_content_filter($wp_content)
{
$my_content = '<p>Hallo World!</p>';
// Add our content before the existing content
$new_content = $my_content . $wp_content;
return $new_content;
}
}
Note that the my_content_filter function was moved inside the class, and now it is instead called from the __construct method.
Also, in order to access the my_content_filter method, we had to slightly modify the add_filter function so that it uses an array instead. The $this variable refers to the object created from the class.
We can also use the add_filter function outside of the class, and then leave out the __construct method. To do this, we would use $name_of_plugin instead of $this:
// Create object from class
$name_of_plugin = new name_of_new_plugin();
// Filters
add_filter('the_content', array($name_of_plugin, 'my_content_filter'));
But, this is not usually the way I do it...
Note. If you are new to object orientated programming, you should check out our tutorials on vanilla PHP: OOP Tutorial - more available here: Object Orientated Programming in PHP
Tell us what you think: