PHP: Object-Oriented Programming Tutorial
Beginners Tutorial in Object-oriented programming in PHP.

Edited: 2020-06-21 13:26
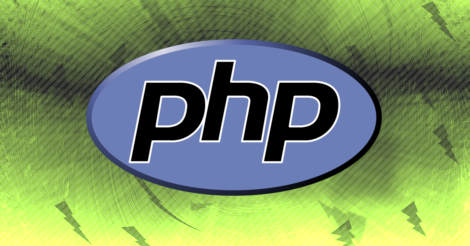
In this tutorial, it will be explained how you can use object-oriented programming in PHP. We will briefly cover how to use classes and objects, so that you will understand what they are before digging into more advanced subjects.
One reason why it is good to use OOP, rather than just basic functions and procedural programming, is to avoid conflicting code. If you had a lot of functions, there will be a high chance that function names would at some point overlap. This is avoided entirely with OOP, and you can easily implement code (libraries) written by other developers, without having to worry about these conflicts.
But, another important benefit, is that you will be forced to use good coding practice, which ultimately makes your code easier to understand for others and yourself. Especially if your application is very large.
Classes and Objects
A class is a template, used to create objects from. Classes can include Properties and Methods (Traditionally known as, variables and functions), which can be used by other functions within the class, or even from the outside.
Variables inside the class can either be declared as public or protected. Public variables can be accessed from everywhere, and protected can only be accessed from within the class itself and by classes that inherits them.
If a property (AKA: variable) is declared using the private keyword, it will only be accessible from within the class.
Properties
When variables are declared inside a class, they will often be referred to as properties. But, often people will also use the variable term. An example of variables being declared in a class can be seen below:
class MyClassName {
public $Property1 = 'Hallo hallo!';
public $MyVariableName = 'Hallo dude!'; // Another Property
}
To access these variables, we first need to create an object from the class, this can be done by writing new when declaring a variable:
$MyObject = new MyClassName(); // Creates an object from the class
We can then output or change the variable values as we wish:
echo $MyObject->Property1 ."<br>";
$MyObject->MyVariableName = 'My House'; // Changes the property value
echo $MyObject->MyVariableName; // Outputs the property value
Methods
When functions are created inside classes, they are referred to as methods. A simple method, which everyone should be able to understand, is shown below:
class MyClassName {
function SayHallo() {
return 'Hallo';
}
}
$MyObject = new MyClassName();
echo $MyObject->SayHallo();
The method simply returns the "hallo" value, and will write "hallo" when used with echo.
Tell us what you think: