How to Get The Window Size With JavaScript
The Width and Height of the browser Window can be obtained through the innerWidth and innerHeight properties; these can be checked by an event handler to detect resize events.
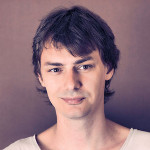
By. Jacob
Edited: 2021-02-13 16:08
Your Window dimensions:
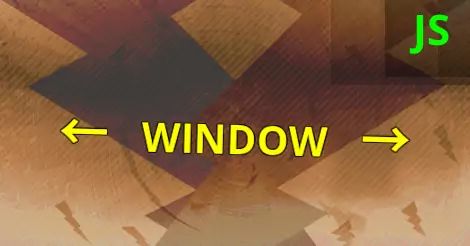
The dimensions of the window can be accessed via the innerWidth and innerHeight properties of the window object; it is important to note that this will only give you the dimensions of the browser Window, and not the screen itself.
Desktop devices allow to resize browser windows, and so, the dimensions of the window object is not going to match the dimensions of the screen. But in most cases, it will probably be sufficient to know the size of the window. To get the current window dimension, you may do like so:
windowWidth = window.innerWidth;
windowHeight = window.innerHeight;
console.log(windowWidth + 'x' + windowHeight);
When people want to detect the screen width, they often want to make changes to the page depending on the available screen real estate; in most cases, it will be better to use CSS media queries for this purpose, but sometimes, design goals can not be achieved exclusively with CSS — this is where it is fine to use JavaScript.
The above example only gives you the current window dimensions, which is useful to detect the window dimensions on the first page load, but not if the user resizes their browser window. To also detect a change in dimensions when a user resizes their browser window, you will have to setup an event listener; this can be done with addEventListener, like so:
let showDimensions = document.querySelector("#show_dimensions");
window.addEventListener('resize', onWindowResize);
function onWindowResize() {
windowWidth = window.innerWidth;
windowHeight = window.innerHeight;
showDimensions.innerHTML = windowWidth + 'x' + windowHeight;
}
This works in all modern browsers. The problem with this approach is that the script can cause lag while people are resizing their browser Window; to avoid lag while resizing, you can make the script less disrupting by adding a setTimeout:
let showDimensions = document.querySelector("#show_dimensions");
let resizeTimer;
window.addEventListener('resize', onWindowResize);
function onWindowResize() {
clearTimeout(resizeTimer);
resizeTimer = setTimeout(function () {
windowWidth = window.innerWidth;
windowHeight = window.innerHeight;
showDimensions.innerHTML = windowWidth + 'x' + windowHeight;
}, 100);
}
Do users resize their browser Window?
Most desktop users will browse the web from a maximized browser window, and this is probably universal, regardless whether they are using Windows, Mac, or Linux; but that does not mean you can just ignore the users who do resize their browser windows.
Occasionally, users will resize their Windows, so it is important you also account for this circumstance, regardless of how rarely it happens. If you do not, then users might experience problems with overlapping elements or elements that cover content when resizing the window.
You will not be able to get the exact screen dimensions of the user, since the browser-UI also takes some space, as well as the taskbar (Aka. system tray or notification area), even when the user maximizes their browser window.
Detect if an element is within the viewport
Now that you know how to obtain the dimensions of the browser window, a natural next step would be to use the innerWidth and innerHeight properties in combination with getBoundingClientRect to detect whether an element is currently in the users view.
The getBoundingClientRect method returns an object that contains the location of the element you are checking. In order for this to work while scrolling, you will need to set an event listner for the scroll event:
let h1 = document.querySelector('h1');
let firstInView = false;
document.addEventListener('scroll', inView);
function inView() {
let bounding = h1.getBoundingClientRect();
if (bounding.top >= 0 && bounding.left >= 0 && bounding.right <= window.innerWidth && bounding.bottom <= window.innerHeight) {
if (false === firstInView) {
console.log('The element was in the view.');
firstInView = true;
}
} else {
console.log('Element not in view');
firstInView = false;
}
}
The firstInView variable ensures that whatever actions you want performed when the element is in the viewport is only performed once, right when the element enters the viewport, and avoids that the code is executed on every single scroll event.
So how is it useful to know if an element is within the view? One place it can be useful is when you want to show a "back to the top" button when the site-footer is within the viewport.
Tell us what you think: