HTTP Location Header
The location header is sent when performing permenant (301) and temporary (302) redirects. In PHP, we can send a Location header using the header function.
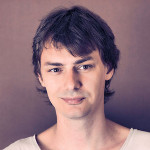
By. Jacob
Edited: 2019-10-13 04:09
The HTTP Location header tells the browser to load another page, often used when performing 301 redirects. Usually a HTTP status code, such as the 303 See Other, is also sent when a location header is used.
The HTTP location header can be sent in response to any request that matches content which has been moved. The browser should automatically pass on the request to the location indicated in this header.
While a relative path might work in some clients, it is recommended to use absolute path with the location header.
$supported_protocols = array(
'HTTP/2.0' => true,
'HTTP/1.1' => true,
'HTTP/1.0' => true,
);
$this->protocol = $_SERVER["SERVER_PROTOCOL"];
if (!isset($supported_protocols["$protocol"])) {
$protocol = 'HTTP/1.0';
}
// Send the Headers
header($protocol . ' 303 See Other');
header('Location: http://beamtic.com/other-page');
Sending headers in PHP
When using the header function, it is a good idea to dynamically determine the protocol in one place, so you will not have to edit all the places the headers are sent in case you later change the protocol.
$protocol = $_SERVER["SERVER_PROTOCOL"];
// Determine the protocol
$supported_protocols = array(
'HTTP/2.0' => true,
'HTTP/1.1' => true,
'HTTP/1.0' => true,
);
$protocol = $_SERVER["SERVER_PROTOCOL"];
if (!isset($supported_protocols["$protocol"])) {
$this->protocol = 'HTTP/1.0';
}
To perform a redirect in PHP, we can use the header function. Assuming we are redirecting to /other-page from the current requested page, we can do like below:
header($protocol . ' 303 See Other');
header('Location: http://beamtic.com/other-page');
Note. Header's should always be sent before any output, such as that produced by echo, print, or by exiting PHP with "?>", otherwise you might get "headers already sent" errors.
Tell us what you think: