The 500 Response Code (Internal Server Error)
The 500 Internal Server Error is usually sent to indicate a problem on the server-side.
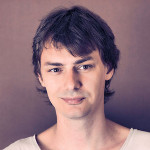
By. Jacob
Edited: 2020-12-01 14:53
A 500 Internal Server Error may be used to indicate that there a problem has happened on the server-side, and that the user did not nessecerily do anything to cause the error.
Servers will often deliver this code automatically, but web developers can also choose to send the error on purpose, which is especially useful when trying to account for various things that can fail.
For example, if we are trying to write to a file, and suddenly the file in not writable, we can choose to log the event for closer inspection, and send the user a 500 Internal Server Error using PHP's http_response_code function.
A 500 response looks like this in plain text:
HTTP/1.1 500 Internal Server Error
To send a custom 500 response code in PHP, use the http_response_code function:
http_response_code(500);
echo '<h1>500 Internal Server Error</h1>';
Sending a raw 500 response
Raw HTTP headers, including status codes, can be sent using PHP's header function, but it is recommended to use http_response_code instead.
If you still want to use the header function, it is recommended you first establish the protocol that the client used in the request, since it must be included when sending raw response codes.
The protocol can be established like this:
function set_protocol() {
$supported_protocols = array(
'HTTP/2.0' => true,
'HTTP/1.1' => true,
'HTTP/1.0' => true,
);
$protocol = $_SERVER["SERVER_PROTOCOL"];
if (!isset($supported_protocols["$protocol"])) {
$protocol = 'HTTP/1.0';
}
return $protocol;
}
This function is also discussed here: PHP: header
Now, if the protocol used by the client was unknown, HTTP/1.0 will be used as a fall-back. You can easily add more protocols as needed. An advantage of using http_response_code is that you do not have to worry about keeping this function updated.
Then, to send the raw response code:
header(set_protocol() . ' 500 Internal Server Error');
echo '<h1>500 Internal Server Error</h1>';
Tell us what you think: