PHP: Obtain the Server IP Address
How to obtain the IP address of the server where the PHP script is running.
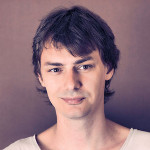
By. Jacob
Edited: 2019-11-03 18:31
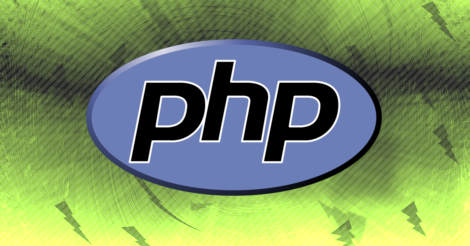
Identifying the external IP address of a server can not be done from PHP natively, and you will therefor have to either use shell_exec or grap it by sending a HTTP request to the website you want to get the IP address from.
We can use the shell_exec function in combination with a regular expression to grep the server's external IP address, to do this, we will call the host command on the local system:
$host_info = shell_exec('nslookup example.com');
preg_match('/^.* ([0-9\:\.]+)\n/', $host_info, $matches);
echo $matches["1"];
This was designed for Windows and Linux systems, and works with IPv4 as well as IPv6. It also works with both the host and nslookup commands. The host command might not be available on Windows.
There are other system commands you can use as well, but nslookup should be installed on most systems.
Get the host name dynamically
If you do not want to hard-code the host name in your script, you can use the HTTP_HOST variable. This will, however, only work when your script is running on a web server. It will not work if you run the script from a terminal.
The below will dynamically obtain the host name:
$host_info = shell_exec('nslookup ' . $_SERVER['HTTP_HOST']);
preg_match('/^.* ([0-9\:\.]+)\n/', $host_info, $matches);
echo $matches["1"];
Do not worry. The HTTP_HOST variable is safe against injection attacks, since it is filled out by the server.
If you attempt to run this script from a terminal, it will most likely result in an error like this:
PHP Notice: Undefined index: HTTP_HOST in /var/www/server_ip.php on line 3
This is because the HTTP_HOST variable is filled out by a web server. When you run PHP from the terminal, $_SERVER variables will not be available.
The $_SERVER super global is an array containing different information made available to us by the web server, and while not all servers will make the same information available, it is generally very reliable.
Obtaining the local server IP
To obtain the local IP address of the server, you can simply echo the contents of the $_SERVER['SERVER_ADDR'] variable. But, again, this will only be available when you run the script on a web server.
echo 'The IP address of the server: ' . $_SERVER['SERVER_ADDR'];
The local IP might be useful for us to know in cases where we only want PHP to respond to requests from a specific network interface.
Obtaining IP addresses
A servers IP (Internet Protocol) address will rarely change, since this can cause interruptions to the web services running on the server. If a website changes its IP address, DNS will have to be updated, and this can be a slow process taking days to finish.
However, the IP of visitors will often change, since ISPs (Internet Service Providers) might be assigning dynamic IPs to their users.
To instead obtain the IP address of a visitor to your website, you may use the REMOTE_ADDR variable:
echo $_SERVER['REMOTE_ADDR'] . PHP_EOL;
A request to the above file should show your IP:
Source: ip.php
If you got a dynamic IP, often all you have to do to get a new IP address is to restart your internet router. In addition to this, some users will also be hiding their real IP address by using proxies or VPNs. This makes it impossible to uniquely identify users based on IP addresses alone.
This does not mean that you should not record IP addresses in your logs. ISPs will usually keep logs, which makes it possible to identify the users using a given IP at a given time. Indeed, some proxies and VPNs also keep logs.
There are ways to try and obtain the real IP behind a proxy or VPN, but they are generally unreliable. So unless you really need to, the best you can do is to just record the IP plainly via $_SERVER['REMOTE_ADDR'].
Another way to obtain the IP address of a website is to simply ping the server from a command prompt or terminal:
ping example.com
PING example.com (93.184.216.34) 56(84) bytes of data.
64 bytes from 93.184.216.34 (93.184.216.34): icmp_seq=1 ttl=53 time=103 ms
...
Tell us what you think: